Kotlin 中的微调器
Android Spinner是一个类似于下拉列表的视图,用于从选项列表中选择一个选项。它提供了一种从项目列表中选择一个项目的简单方法,当我们单击它时,它会显示所有值的下拉列表。
android spinner 的默认值将是当前选择的值,通过使用Adapter ,我们可以轻松地将项目绑定到 spinner 对象。
通常,我们通过在 Kotlin 文件中使用ArrayAdapter来使用项目列表填充 Spinner 控件。
首先,我们按照以下步骤创建一个新项目:
- 单击File ,然后单击New => New Project 。
- 之后包括 Kotlin 支持,然后单击下一步。
- 根据方便选择最小的 SDK,然后单击下一步按钮。
- 然后选择Empty activity => next => finish 。
Spinner 小部件的不同属性
XML attributes | Description |
---|---|
android:id | Used to specify the id of the view. |
android:textAlignment | Used to the text alignment in the dropdown list. |
android:background | Used to set the background of the view. |
android:padding | Used to set the padding of the view. |
android:visibilty | Used to set the visibility of the view. |
android:gravity | Used to specify the gravity of the view like center, top, bottom etc |
修改activity_main.xml文件
在此文件中,我们使用 TextView 和 Spinner 小部件并设置它们的属性。
XML
XML
SpinnerInKotlin
Selected item:
- Java
- Kotlin
- Swift
- Python
- Scala
- Perl
Kotlin
package com.geeksforgeeks.myfirstkotlinapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.View
import android.widget.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// access the items of the list
val languages = resources.getStringArray(R.array.Languages)
// access the spinner
val spinner = findViewById(R.id.spinner)
if (spinner != null) {
val adapter = ArrayAdapter(this,
android.R.layout.simple_spinner_item, languages)
spinner.adapter = adapter
spinner.onItemSelectedListener = object :
AdapterView.OnItemSelectedListener {
override fun onItemSelected(parent: AdapterView<*>,
view: View, position: Int, id: Long) {
Toast.makeText(this@MainActivity,
getString(R.string.selected_item) + " " +
"" + languages[position], Toast.LENGTH_SHORT).show()
}
override fun onNothingSelected(parent: AdapterView<*>) {
// write code to perform some action
}
}
}
}
}
XML
更新字符串.xml 文件
在这里,我们使用字符串标签更新应用程序的名称。我们还创建将在下拉菜单中使用的项目列表。
XML
SpinnerInKotlin
Selected item:
- Java
- Kotlin
- Swift
- Python
- Scala
- Perl
在 MainActivity.kt 文件中访问 Spinner
首先,我们声明一个变量语言来访问字符串.xmnl 文件中的字符串项。
val languages = resources.getStringArray(R.array.Languages)
然后,我们访问微调器并设置ArrayAdaptor来控制项目列表。
val spinner = findViewById(R.id.spinner)
if (spinner != null) {
val adapter = ArrayAdapter(this,
android.R.layout.simple_spinner_item, languages)
spinner.adapter = adapter
科特林
package com.geeksforgeeks.myfirstkotlinapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.View
import android.widget.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// access the items of the list
val languages = resources.getStringArray(R.array.Languages)
// access the spinner
val spinner = findViewById(R.id.spinner)
if (spinner != null) {
val adapter = ArrayAdapter(this,
android.R.layout.simple_spinner_item, languages)
spinner.adapter = adapter
spinner.onItemSelectedListener = object :
AdapterView.OnItemSelectedListener {
override fun onItemSelected(parent: AdapterView<*>,
view: View, position: Int, id: Long) {
Toast.makeText(this@MainActivity,
getString(R.string.selected_item) + " " +
"" + languages[position], Toast.LENGTH_SHORT).show()
}
override fun onNothingSelected(parent: AdapterView<*>) {
// write code to perform some action
}
}
}
}
}
AndroidManifest.xml 文件
XML
作为模拟器运行:
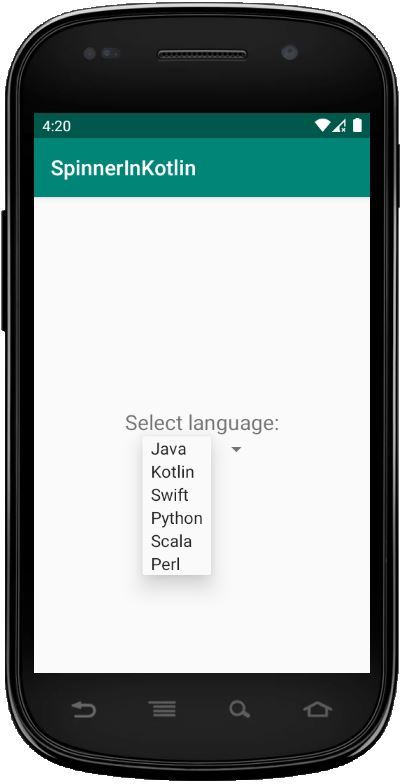
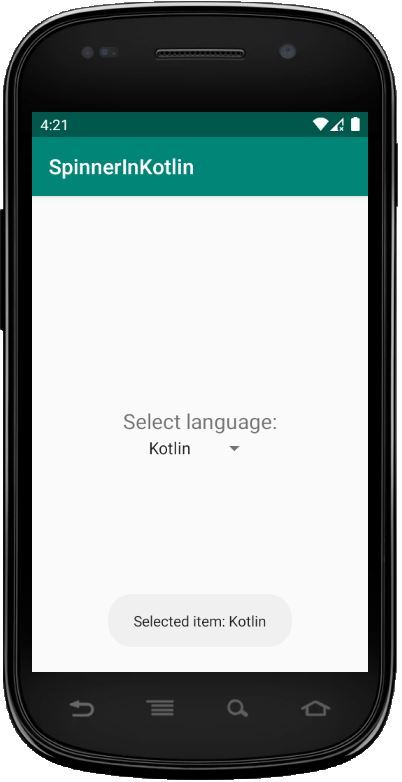
在评论中写代码?请使用 ide.geeksforgeeks.org,生成链接并在此处分享链接。