Python MongoDB – Update_many 查询
MongoDB是一个 NoSQL 数据库管理系统。与 MySQL 不同,MongoDB 中的数据不存储为关系或表。 mongoDB 中的数据存储为文档。文档是类似 Javascript/JSON 的对象。 MongoDB 中更正式的文档使用 BSON。 PyMongo是一个用于Python的 MongoDB API。它允许使用Python脚本从 MongoDB 数据库中读取和写入数据。它需要在系统上安装Python和 mongoDB。
更新_many()
更新函数已在较新版本的 MongoDB(3.xx 及更高版本)中被弃用。较早的更新函数可用于单次更新和使用“multi = true”的多次更新。但在较新版本的 mongoDB 中,建议使用 update_many() 和 update_one()。
主要区别在于,如果查询要更新单个或多个文档,用户需要提前计划。
句法:
db.collection.updateMany(
,
,
{
upsert: ,
writeConcern: ,
collation: ,
arrayFilters: [ , ... ],
hint:
}
)
MongoDB 中的更新运算符
设定值:
- $set:用于设置字段值。
- $setOnInsert:仅在插入新文档时更新值。
- $unset:删除字段及其值。
数字运算符:
- $inc:将值增加给定的数量。
- $min/$max:返回最小值或最大值。
- $mul:将值乘以给定的数量。
杂项运算符:
- $currentDate:将字段的值更新为当前日期。
- $rename : 重命名一个字段
样本数据库:
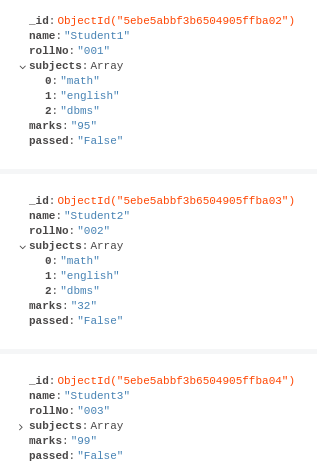
我们将在本文中看到一些用例,其中更新许多记录可能很有用:
- 根据条件更改或增加多个元素。
- 将新字段插入多个或所有文档。
示例 1:所有分数大于 35 的学生都通过了。
Python3
from pymongo import MongoClient
# Creating an instance of MongoClient
# on default localhost
client = MongoClient('mongodb://localhost:27017')
# Accessing desired database and collection
db = client.gfg
collection = db["classroom"]
# Update passed field to be true for all
# students with marks greater than 35
collection.update_many(
{"marks": { "$gt": "35" } },
{
"$set": { "passed" : "True" }
}
)
Python
from pymongo import MongoClient
# Creating an instance of MongoClient
# on default localhost
client = MongoClient('mongodb://localhost:27017')
# Accessing desired database and collection
db = client.gfg
collection = db["classroom"]
# Address filed to be added to all documents
collection.update_many(
{},
{"$set":
{
"Address": "value"
}
},
# don't insert if no document found
upsert=False,
array_filters=None
)
查询后的数据库:
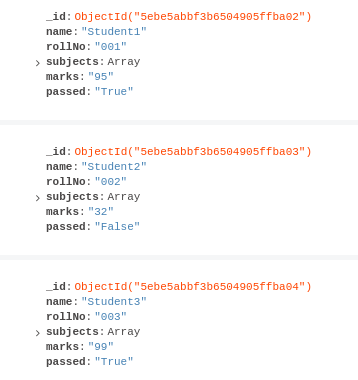
示例 2:将名为地址的新字段添加到所有文档
Python
from pymongo import MongoClient
# Creating an instance of MongoClient
# on default localhost
client = MongoClient('mongodb://localhost:27017')
# Accessing desired database and collection
db = client.gfg
collection = db["classroom"]
# Address filed to be added to all documents
collection.update_many(
{},
{"$set":
{
"Address": "value"
}
},
# don't insert if no document found
upsert=False,
array_filters=None
)
数据库查询后:
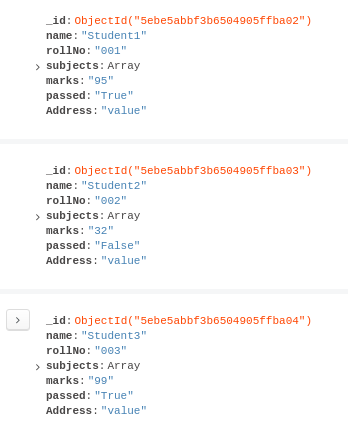