使用Java缩小 PDF 中的内容
用于缩小 PDF 文档内容的程序。程序中需要导入外部jar文件。下面是相同的实现。
方法:
1. 创建一个空的 PDF 文件。
- 将空 PDF 的路径分配给 String 变量。
- 从包com.itextpdf.kernel.pdf导入PdfWriter 。 (PdfWriter 允许我们在 PDF 文件上写入内容)
- PdfWriter 接受一个字符串变量作为它的参数,它表示 PDF 的目标。
- 通过传递 PDF 文件的路径来初始化 PdfWriter 对象。
2. 创建一个代表空 PDF 文件的文档。
- 从包com.itextpdf.kernel.pdf 导入 PdfDocument 。 (PdfDocument 用于表示代码中的 pdf。此后可用于添加或修改各种功能,例如字体、图像等)
- PdfDocument 接受 PdfWriter 或 PdfReader 对象作为它的参数。
- 通过传递 PdfWriter 对象来初始化 PdfDocument。
3.对原始PDF重复上述步骤。
- 将原始 PDF 的路径分配给 String 变量。
- 从包com.itextpdf.kernel.pdf 导入 PdfReader 。 (PdfReader 允许我们阅读 PDF 文件中的内容)
- 将原始 PDF 的路径传递给 PdfReader 构造函数。
- 通过传递 PdfReader 对象来初始化 PdfDocument。
4.从原始PDF获取页面大小。
- 从包com.itextpdf.kernel.pdf 导入 PdfPage 。 (PdfPage 代表 PDF 中的特定页面)
- 从包com.itextpdf.kernel.geom导入Rectangle 。
- PdfDocumen t类中存在的方法getPage(int pageNumber)返回指定特定页面的 PdfPage 对象。
- PdfPage 类中的getPageSize()方法返回特定 PdfPage 对象的 Rectangle 对象。
5.从空PDF中获取页面大小。
- 无法从空 PDF 获取页面大小。
- 因此,为了获得大小,我们使用 PdfDocument 类中的addNewPage()方法向空 PDF 添加一个新页面。 addNewPage() 方法返回一个 PdfPage 对象。
- PdfPage 类中的getPageSize()方法返回特定 PdfPage 对象的 Rectangle 对象。
6. 创建原始页面的缩小版本。
- 从包com.itextpdf.kernel.geom导入AffineTransform 。
- 可以使用 (emptyPageWidth/originalPageWidth)/2 计算新的缩放宽度。
- 可以使用 (emptyPageHeight/originalPageHeight)/2 计算新的缩放高度。
- AffineTransform 类中存在的静态方法getScaleInstance(double width, double height)返回具有缩放宽度和缩放高度的 AffineTransform 对象。
7. 将原始页面的缩小版本附加到空 PDF。
- 从包com.itextpdf.kernel.pdf.canvas 导入 PdfCanvas 。
- 通过传入空页面作为参数来初始化 PdfCanvas 对象。
- 将上面创建的缩小尺寸的矩阵添加到空画布。
- 使用方法copyAsFormXObject(PdfDocument shrunkenDocument)从原始页面复制内容(返回一个 PdfFormXObject) 出现在 PdfPage 类中。
- 使用addXObject(PdfXObject xObject, float x, float y) 方法将复制的页面添加到画布。
8. 创建文档。
- 从包com.itextpdf.layout导入文档。
- 创建一个 Document 对象来制作 PDF 的可读版本。
- Document 类的构造函数之一接受 PdfDocument 对象作为它的参数。
- 通过传递 shrunkenDocument 作为参数来初始化 Document 对象。
- 创建对象后关闭文档以防止内存泄漏。
注意:需要外部 Jar(点击此处下载)。
下面是PDF缩小的实现:
Java
// Java program to shrink the contents of a PDF
// Importing the necessary libraries required
import com.itextpdf.kernel.pdf.*;
import com.itextpdf.kernel.geom.Rectangle;
import com.itextpdf.kernel.geom.AffineTransform;
import com.itextpdf.kernel.pdf.canvas.PdfCanvas;
import com.itextpdf.kernel.pdf.xobject.PdfFormXObject;
import com.itextpdf.layout.Document;
public class Main {
public static void main(String[] args)
{
// Try catch block is used to handle File Exceptions
try {
// Destination of the empty PDF
String shrunkenPath = "/home/mayur/newGFG.pdf";
// Creating PDF writer object
PdfWriter pdfWriter
= new PdfWriter(shrunkenPath);
// Creating a PdfDocument object for empty pdf
PdfDocument shrunkenDocument
= new PdfDocument(pdfWriter);
// Destination of the original PDF
String originalPath = "/home/mayur/GFG.pdf";
// Creating PDF reader object
PdfReader pdfReader
= new PdfReader(originalPath);
// Creating a PdfDocument object for original
// pdf
PdfDocument originalDocument
= new PdfDocument(pdfReader);
// Opening the first page of the original PDF
PdfPage orignalPage
= originalDocument.getPage(1);
// Getting the height and width of the original
// PDF
Rectangle originalPDFSizes
= orignalPage.getPageSize();
// Adding a new page to the empty PDF
PdfPage emptyPage
= shrunkenDocument.addNewPage();
// Getting the height and width of the empty PDF
Rectangle emptyPDFsizes
= emptyPage.getPageSize();
// Scaling down the original Pdf page
double width = emptyPDFsizes.getWidth()
/ originalPDFSizes.getWidth();
double height = emptyPDFsizes.getHeight()
/ originalPDFSizes.getHeight();
// Calculating the new width and height
double newWidth = width / 2;
double newHeight = height / 2;
// Creating a matrix with new width and new
// height
AffineTransform affineTransform
= AffineTransform.getScaleInstance(
newWidth, newHeight);
// Creating an empty canvas
PdfCanvas canvas = new PdfCanvas(emptyPage);
// Adding the matrix created to the empty canvas
canvas.concatMatrix(affineTransform);
// Copying the content from the original PDF
PdfFormXObject pageCopy
= orignalPage.copyAsFormXObject(
shrunkenDocument);
// Adding the copied page to the canvas
canvas.addXObject(pageCopy, (float)newWidth,
(float)newHeight);
// Creating a Document object to make the PDF
// readable
Document doc = new Document(shrunkenDocument);
// Closing the documents to prevent memory leaks
doc.close();
originalDocument.close();
System.out.println(
"Shrunken PDF successfully created");
}
// Catching any unwanted Exceptions
catch (Exception e) {
System.err.println(e);
}
}
}
执行前:
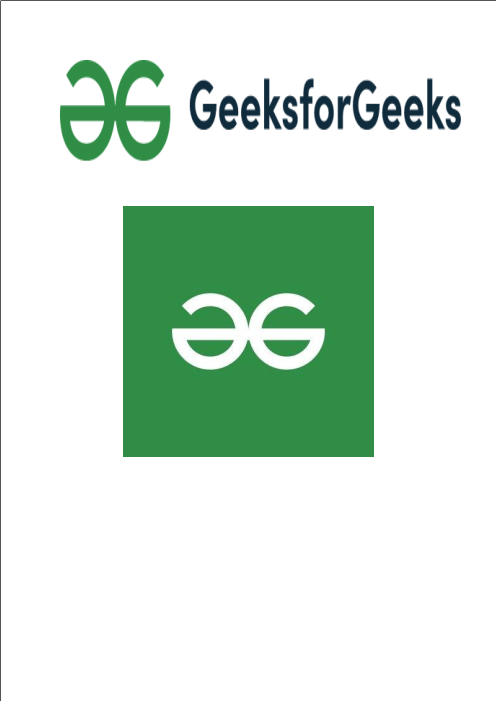
原始PDF
执行后:
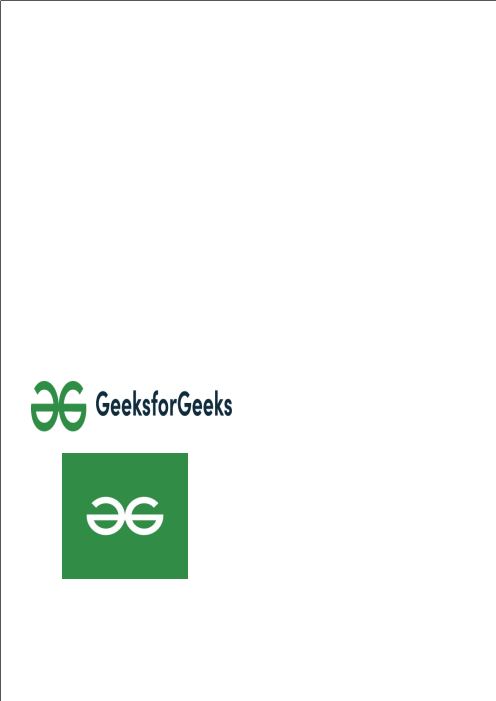
内容缩小后的 PDF