使用语音识别重新启动计算机
我们可以在Python的帮助下做到这一点。 Python有很多库可以帮助很多事情变得容易。我们需要终端的帮助来完成这项任务。 Python 最好的库之一语音识别将帮助我们做到这一点。
所需模块
- Pyttsx3:这是Python中的文本到语音库。我们必须使用pip。
pip install pyttsx3
- 语音识别模块:该模块将帮助我们识别给计算机的语音和命令。我们必须使用 pip 来安装语音识别。
pip install SpeechRecognition
- OS模块: Python中提供与操作系统交互函数的模块。 OS 模块随Python包一起提供。点子不是必需的。
为什么使用终端?
当我们在终端中键入 shutdown 时,我们将获得许多选项,借助这些选项,我们可以重新启动计算机。因为我们将有很多标签。我们可以使用“/r”和关闭来重新启动。
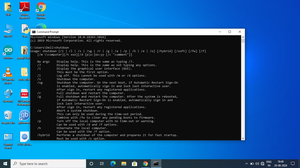
带有关机命令的终端。我们可以看到 /r 表示完全关闭和重新启动。
循序渐进的方法:
步骤 1:我们必须使 Speak函数使计算机与我们进行通信。
Python3
# Method to give
# output voice commands
def Speak(self, audio):
engine = pyttsx3.init('sapi5')
# initial constructor of pyttsx3
voices = engine.getProperty('voices')
# getter and setter method
engine.setProperty('voice', voices[1].id)
engine.say(audio)
engine.runAndWait()
Python3
# Method to take
# input voice commands
def take_commands(self):
r = sr.Recognizer()
# Making the use of Recognizer
# and Microphone method from
# Speech Recognition for taking commands
with sr.Microphone() as source:
print('Listening')
r.pause_threshold = 0.7
# seconds of non-speaking audio
# before a phrase is considered complete
audio = r.listen(source)
try:
print("Recognizing")
Query = r.recognize_google(audio, language='en-in')
# For listening the command in indian english
print("the query is printed='", Query, "'")
# For printing the query or the
# command that we give
except Exception as e:
# This is for printing the exception
print(e)
print("Say that again sir")
return "None"
return Query
Python3
# Method to restart PC
def restart(self):
self.Speak("do u want to restart the computer sir")
take = self.takeCommand()
choice = take
if choice == 'yes':
print("Restarting the computer")
self.Speak("Restarting the computer")
os.system("shutdown /r /t 30")
if choice == 'no':
print("Thank u sir")
self.Speak("Thank u sir")
Python3
# Driver Code
if __name__ == '__main__':
Maam = Main()
Maam.restart()
Python3
# Import required modules
import pyttsx3
import time
import speech_recognition as sr
import os
# Creating class
class Main:
# Method to give output commands
def Speak(self, audio):
engine = pyttsx3.init('sapi5')
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[1].id)
engine.say(audio)
engine.runAndWait()
# Method to take input voice commands
def takeCommand(self):
# This method is for taking
# the commands and recognizing the command
r = sr.Recognizer()
# from the speech_Recognition module
# we will use the recongizer method
# for recognizing
with sr.Microphone() as source:
# from the speech_Recognition module
# we will use the Microphone module for
# listening the command
print('Listening')
# seconds of non-speaking audio
# before a phrase is considered complete
r.pause_threshold = 0.7
audio = r.listen(source)
try:
print("Recognizing")
Query = r.recognize_google(audio, language='en-in')
# for listening the command
# in indian english
print("the query is printed='", Query, "'")
# for printing the query or the
# command that we give
except Exception as e:
# this method is for handling
# the exception and so that
# assistant can ask for telling
# again the command
print(e)
print("Say that again sir")
return "None"
return Query
# Method to restart PC
def restart(self):
self.Speak("do u want to switch off the computer sir")
take = self.takeCommand()
choice = take
if choice == 'yes':
print("Shutting down the computer")
os.system("shutdown /s /t 30")
self.Speak("Shutting the computer")
if choice == 'no':
print("Thank u sir")
self.Speak("Thank u sir")
# Driver Code
if __name__ == '__main__':
Maam = Main()
Maam.restart()
第 2 步:我们现在必须创建一个函数来接收我们的命令。
蟒蛇3
# Method to take
# input voice commands
def take_commands(self):
r = sr.Recognizer()
# Making the use of Recognizer
# and Microphone method from
# Speech Recognition for taking commands
with sr.Microphone() as source:
print('Listening')
r.pause_threshold = 0.7
# seconds of non-speaking audio
# before a phrase is considered complete
audio = r.listen(source)
try:
print("Recognizing")
Query = r.recognize_google(audio, language='en-in')
# For listening the command in indian english
print("the query is printed='", Query, "'")
# For printing the query or the
# command that we give
except Exception as e:
# This is for printing the exception
print(e)
print("Say that again sir")
return "None"
return Query
第 3 步:现在我们将制作一个 Restart 方法来使计算机重新启动。
蟒蛇3
# Method to restart PC
def restart(self):
self.Speak("do u want to restart the computer sir")
take = self.takeCommand()
choice = take
if choice == 'yes':
print("Restarting the computer")
self.Speak("Restarting the computer")
os.system("shutdown /r /t 30")
if choice == 'no':
print("Thank u sir")
self.Speak("Thank u sir")
第 4 步:现在我们将拥有 main 方法。
蟒蛇3
# Driver Code
if __name__ == '__main__':
Maam = Main()
Maam.restart()
以下是上述方法的完整程序:
蟒蛇3
# Import required modules
import pyttsx3
import time
import speech_recognition as sr
import os
# Creating class
class Main:
# Method to give output commands
def Speak(self, audio):
engine = pyttsx3.init('sapi5')
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[1].id)
engine.say(audio)
engine.runAndWait()
# Method to take input voice commands
def takeCommand(self):
# This method is for taking
# the commands and recognizing the command
r = sr.Recognizer()
# from the speech_Recognition module
# we will use the recongizer method
# for recognizing
with sr.Microphone() as source:
# from the speech_Recognition module
# we will use the Microphone module for
# listening the command
print('Listening')
# seconds of non-speaking audio
# before a phrase is considered complete
r.pause_threshold = 0.7
audio = r.listen(source)
try:
print("Recognizing")
Query = r.recognize_google(audio, language='en-in')
# for listening the command
# in indian english
print("the query is printed='", Query, "'")
# for printing the query or the
# command that we give
except Exception as e:
# this method is for handling
# the exception and so that
# assistant can ask for telling
# again the command
print(e)
print("Say that again sir")
return "None"
return Query
# Method to restart PC
def restart(self):
self.Speak("do u want to switch off the computer sir")
take = self.takeCommand()
choice = take
if choice == 'yes':
print("Shutting down the computer")
os.system("shutdown /s /t 30")
self.Speak("Shutting the computer")
if choice == 'no':
print("Thank u sir")
self.Speak("Thank u sir")
# Driver Code
if __name__ == '__main__':
Maam = Main()
Maam.restart()