HAUS Connect – Python项目
大学生面临的最常见问题之一是我们的时间表非常不稳定,跟上时间表是一项繁琐的任务,在每个人都独自一人的大流行时期,这些问题被极大地放大了。此应用程序的目的是帮助学生跟踪他们的课程、测试和作业,并协助简化教师与学生之间的沟通。 HAUS-Connect 是一个供大学教师安排或重新安排会议或讲座的平台,他们也可以设置考试提醒和上传学习资料。聊天机器人将帮助寻找学习材料。基本上,我们希望确保我们每个人都不会错过任何截止日期。目前这是针对大学生的,但它的多功能性是巨大的,它可以大规模用于整个企业的通信,一个管理休假、休假余额、待处理工作等的单一系统。
特征
- 教师可以上传录制的讲座链接、.ppt 文件、参考书pdf。
- 时间表修改访问将只提供给教职员工。
- 将提供一个疑问部分,学生必须上传他的疑问图片并选择主题,教师屏幕上将弹出一条消息,他可以进一步发送解决方案。
- 聊天机器人将帮助寻找学习材料
- 如果教师重新安排特定会议,将直接向所有学生发送短信和电子邮件。
使用的工具:
客户端 :
- html
- CSS
- javascript
服务器端 :
- MySQL数据库
- Python
- 短信接口
- 姜戈
说明图
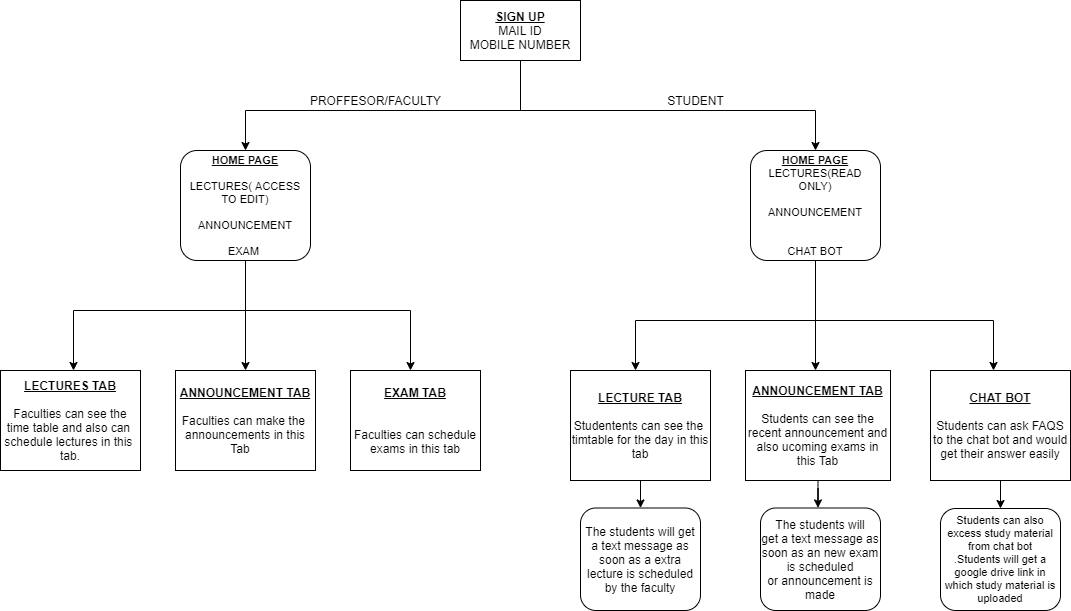
说明图
登录图
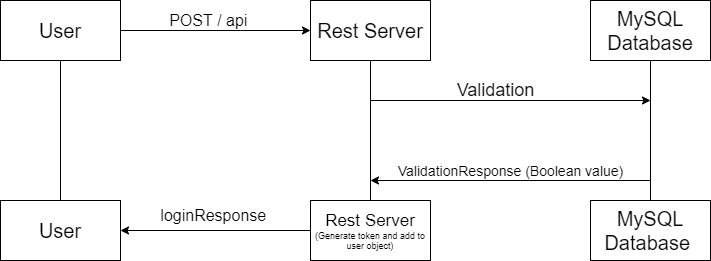
登录图
代码流
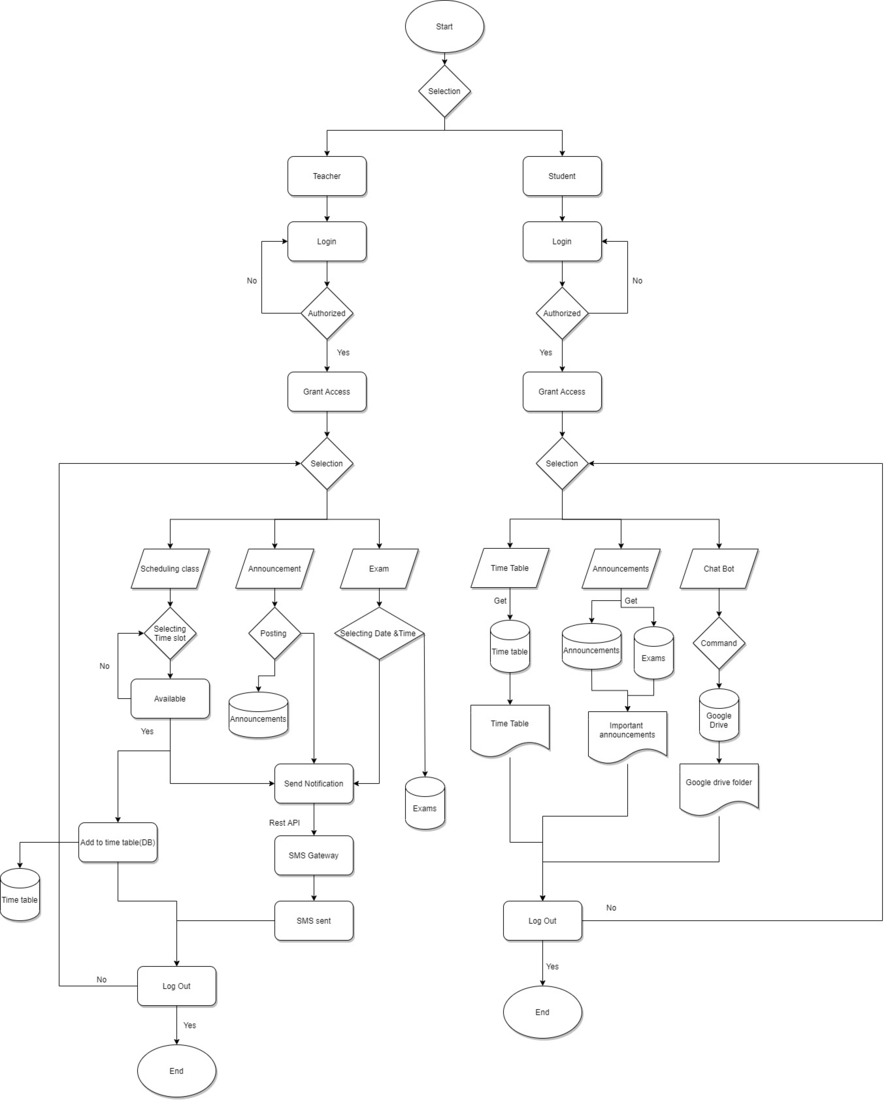
代码流
分步实施
Broadcaster:这是包含主项目文件的文件夹。
设置.py
此文件声明所有依赖项和其他设置。
Python3
from pathlib import Path
import os
# Build paths inside the project like this:
# BASE_DIR / 'subdir'.
BASE_DIR = Path(__file__).resolve().parent.parent
# SECURITY WARNING: keep the secret key used in
# production secret!
SECRET_KEY = 'django-insecure-lzy=xp#!(0e-h9+%u!dsj$m4-2+=j7r$5hsb((@7ziv2g=dgbt'
# SECURITY WARNING: don't run with debug turned
# on in production!
DEBUG = True
ALLOWED_HOSTS = []
# Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'Teacher.apps.TeacherConfig',
'Student.apps.StudentConfig',
'Register.apps.RegisterConfig',
'crispy_forms',
]
MIDDLEWARE = [
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
]
ROOT_URLCONF = 'broadcaster.urls'
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
WSGI_APPLICATION = 'broadcaster.wsgi.application'
# Database
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': BASE_DIR / 'db.sqlite3',
}
}
# Password validation
AUTH_PASSWORD_VALIDATORS = [
{
'NAME': 'django.contrib.auth.password_validation.\
UserAttributeSimilarityValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.\
MinimumLengthValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.\
CommonPasswordValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.\
NumericPasswordValidator',
},
]
# Internationalization
LANGUAGE_CODE = 'en-us'
TIME_ZONE = 'UTC'
USE_I18N = True
USE_L10N = True
USE_TZ = True
# Static files (CSS, JavaScript, Images)
STATIC_URL = '/static/'
STATIC_ROOT = os.path.join(BASE_DIR, 'static')
CRISPY_TEMPLATE_PACK = "bootstrap4"
LOGIN_REDIRECT_URL = "/"
# Default primary key field type
DEFAULT_AUTO_FIELD = 'django.db.models.BigAutoField'
Python3
from django.contrib import admin
from django.urls import path, include
from Register import views as v
urlpatterns = [
path('admin/', admin.site.urls),
path('', include("Teacher.urls")),
path('', include("Student.urls")),
path('register/', v.register, name="register"),
path("", include("django.contrib.auth.urls")),
]
HTML
{%load static%}
{% load crispy_forms_tags %}
Login/SignUp
{{form.errors}}
HTML
{%load static%}
{% if user.is_authenticated %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
You are already logged in
Click here if you are a professor
Click here if you are a student
{% else %}
{% load crispy_forms_tags %}
Login/SignUp
{{form.errors}}
Dont have an account? create one here
{% endif %}
Python3
from django.apps import AppConfig
class RegisterConfig(AppConfig):
default_auto_field = 'django.db.models.BigAutoField'
name = 'Register'
Python3
from django import forms
from django.contrib.auth import login, authenticate
from django.contrib.auth.forms import UserCreationForm
from django.contrib.auth.models import User
class RegistrationForm(UserCreationForm):
email = forms.EmailField()
class Meta:
model = User
# order in which the fields show up
fields = ["email", "username", "password1", "password2"]
Python3
from django.shortcuts import render,redirect
from django.contrib.auth import login,authenticate,logout
from .forms import RegistrationForm
# Create your views here.
def register(request):
if request.user.is_authenticated:
return redirect("/")
if request.method == 'POST':
form=RegistrationForm(request.POST)
if form.is_valid():
form.save()
return redirect('login')
else:
form=RegistrationForm()
return render(request,"Register/register.html",{"form":form})
HTML
{% load static %}
{% if user.is_authenticated %}
About Us
HAUS Developers
- Hardeep
- Ahmed
- Utsav
- Sarthak
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
ChatBot
ChatBot
Hi I am Era
Under Construction
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
FAQ
Frequently Asked Questions
Why shouldn't we trust atoms?
They make up everything
What do you call someone with no body and no nose?
Nobody knows.
What's the object-oriented way to become wealthy?
Inheritance.
How many tickles does it take to tickle an octopus?
Ten-tickles!
What is: 1 + 1?
Depends on who are you asking.
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
Student's Portal
HAUS-Connect
Student's Portal
HAUS-Connect is platform where all the faculties can send notice,Schedule Exam and Schedule Lectures. Any action performed by faculty will send a text message to all the students informing them about any upcoming events. HAUS-Connect aims to decrease the ridge between Student and Faculty.It is a firm of the Students,by the Students, for the Students.
Announcement
{% for t in t1 %}
{{ t }}
{% endfor %}
Lectures
{% for t in t2 %}
You have a {{t.subject}}
extra class on {{t.date}}
from {{t.time_start}}
to {{t.time_end}}
{% endfor %}
Exam
{% for t in t3 %}
You have a {{t.subject}}
extra class on {{t.date}}
from {{t.time_start}}
to {{t.time_end}}
{% endfor %}
© 2021 HAUS - All Rights Reserved
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
TIMETABLE
Sunday
Monday
Tuesday
Wednesday
Thursday
Friday
Saturday
8:00 AM - 9:00 AM
--
Data Structures
Digital Electronics
Microprocessor Programming
OOPS With Java
Open Elective
--
9:00 AM - 10:00 AM
--
Discrete Math
Discrete Math
OOPS With Java
Data Structures
Data Structures - Lab
--
10:00 AM - 11:00 AM
--
Open Elective
Data Structures - Lab
Data Structures
Discrete Math
OOPS With Java
--
11:00 AM - 12:00 PM
--
Microprocessor Programming - Lab
OOPS With Java
Open Elective
Digital Electronics - Lab
Microprocessor Programming
--
12:00 PM - 1:00 PM
--
Digital Electronics
Microprocessor Programming
Discrete Math - Tutorial
Microprocessor Programming - Lab
OOPS With Java - Lab
--
1:00 PM - 2:00 PM
--
Discrete Math
OOPS With Java - Lab
Data Structures
Digital Electronics
Discrete Math
--
2:00 PM - 3:00 PM
--
--
--
--
--
--
--
3:00 PM - 4:00 PM
--
--
--
--
--
--
--
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
Python3
from Teacher.models import Announcements, Exam, Class
from django.shortcuts import render
from django.contrib.auth import logout
from django.http import HttpResponse, HttpResponseRedirect
# Create your views here.
def home(response):
t1 = Announcements.objects.all()
t2 = Class.objects.all()
t3 = Exam.objects.all()
return render(response, "Student/student_home.html",
{"t1": t1, "t2": t2, "t3": t3})
def chatbot(response):
return render(response, "Student/ChatBot.html")
def timetable(response):
return render(response, "Student/TimeTable.html")
def result(response):
return render(response, "Student/Result.html")
def faq(response):
return render(response, "Student/faq.html")
def logout_request(request):
logout(request)
return render(request, "Student/student_home.html", {})
def about(request):
return render(request, "Student/About.html", {})
Python3
from django.urls import path
from . import views
urlpatterns = [
path("student_home/", views.home, name="Home"),
path("chatbot/", views.chatbot, name="ChatBot"),
path("FAQ/", views.faq, name="FAQ"),
path("Result/", views.result, name="Result"),
path("TimeTable/", views.timetable, name="TimeTable"),
path("logout/", views.logout_request, name="logout"),
path("about/", views.about, name="About")
]
HTML
{% load static %}
{% if user.is_authenticated %}
Create Announcement
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
You dont have authorization to access this page.
If you are a professor contact administrator.
Click here if you are a student
HTML
{% load static %}
{% if user.is_authenticated %}
Schedule Class
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
Schedule Exam
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
Teacher's Portal
{% include 'Teacher/messages.html' %}
HAUS-Connect
Teacher's Portal
Basically, here all Faculty's can send notice , Schedule Exam and Schedule Lectures. Any action performed by Faculty will send a text message to all students informing them about any upcoming events. HAUS-Connect aims to decrease the ridge between Student and Faculty.It is a firm of the Students,by the Students, for the Students.
TimeTable
*Note this is fixed timetable provided by the institution
We the students of PDEU CE`20 batch, appreciate you for putting all your efforts and helping us throughout the day from solving our 'silly' doubts to help us in any problem which we faced during college.
-Thanking You.
© 2021 HAUS - All Rights Reserved
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
Login here
{% load crispy_forms_tags %}
Register Here
HTML
{% if messages %}
{% for message in messages %}
{% endfor %}
{% endif %}
Python3
from django.contrib import admin
from .models import *
# Register your models here.
admin.site.register(Announcements)
admin.site.register(Class)
admin.site.register(Exam)
Python3
from django.apps import AppConfig
class TeacherConfig(AppConfig):
default_auto_field = 'django.db.models.BigAutoField'
name = 'Teacher'
Python3
from django.http import HttpResponse
from django.shortcuts import redirect, render
def allowed_users(allowed_roles=[]):
def decorator(view_func):
def wrapper_func(request, *args, **kwargs):
group = None
if request.user.groups.exists():
group = request.user.groups.all()[0].name
if group in allowed_roles:
return view_func(request, *args, **kwargs)
else:
return render(request, "Teacher/base.html", {})
return wrapper_func
return decorator'
Python3
from django import forms
class createnewannouncement(forms.Form):
text = forms.CharField(label="Announcement", max_length=1000)
check = forms.BooleanField(label="Send message", required=False)
class schedule_extra_class(forms.Form):
subject = forms.CharField(label="Subject", max_length=20)
date = forms.CharField(label="Date", max_length=20)
time_start = forms.CharField(label="Starting Time", max_length=20)
time_end = forms.CharField(label="Ending Time", max_length=20)
check = forms.BooleanField(label="Send message", required=False)
class schedule_exam(forms.Form):
subject = forms.CharField(label="Subject ", max_length=20)
date = forms.CharField(label="Date", max_length=20)
time_start = forms.CharField(label="Starting Time", max_length=20)
time_end = forms.CharField(label="Ending Time", max_length=20)
check = forms.BooleanField(label="Send message", required=False)
Python3
from django.db import models
class Announcements(models.Model):
text = models.TextField(max_length=500)
def __str__(self):
return self.text
class Class(models.Model):
subject = models.TextField(max_length=50)
date = models.TextField(max_length=50)
time_start = models.TextField(max_length=5)
time_end = models.TextField(max_length=5)
message = "You have a {0} extra class on {1} from {2} to {3}".format(
str(subject), str(date), str(time_start), str(time_end))
def __str__(self):
message = "You have a {0} extra class on {1} from {2} to {3}".format(
str(self.subject), str(self.date), str(self.time_start), str(self.time_end))
return self.message
class Exam(models.Model):
subject = models.TextField(max_length=50)
date = models.TextField(max_length=50)
time_start = models.TextField(max_length=5)
time_end = models.TextField(max_length=5)
message = "You have a {0} exam on {1} from {2} to {3}".format(
str(subject), str(date), str(time_start), str(time_end))
def __str__(self):
message = "You have a {0} exam on {1} from {2} to {3}".format(
str(self.subject), str(self.date), str(self.time_start), str(self.time_end))
return self.message
Python3
def send_message(message):
from twilio.rest import Client
client = Client("Account SId", "Auth token")
numbers = ["+916351816925", "+91xxxxxxxxxx",
"+91xxxxxxxxxx", "+91xxxxxxxxxx"]
names = ["Sarthak", "Hardeep", "Utsav", "Ahmed"]
for i in range(4):
m = ("Hello {} ".format(names[i]))+message
client.messages.create(to=numbers[i],
from_="number provided by the api",
body=(m))
Python3
from django.urls import path
from . import views
urlpatterns = [
path("", views.home, name="Home"),
path("", views.index, name="index"),
path("announcement/", views.create_announcement, name="Announcement"),
path("class/", views.create_class, name="Extra Class"),
path("exam/", views.create_exam, name="Exams"),
path("logout/", views.logout_request, name="logout"),
path("base/", views.base, name="Base"),
]
Python3
from datetime import date
from django.shortcuts import render
from django.contrib.auth import logout
from django.http import HttpResponse, HttpResponseRedirect
from django.contrib import messages
from .decorator import allowed_users
from .models import *
from .forms import *
from .Sending_messages import *
def base(request):
return render(request, "Teacher/base.html")
@allowed_users(allowed_roles=['admin', 'Professor'])
def home(response):
return render(response, "Teacher/home.html")
def logout_request(request):
logout(request)
return render(request, "Teacher/home.html", {})
@allowed_users(allowed_roles=['admin', 'Professor'])
def index(response, id):
a = Announcements.objects.get(id=id)
return render(response, "Teacher/home.html", {})
@allowed_users(allowed_roles=['admin', 'Professor'])
def create_announcement(response):
if response.method == "POST":
form = createnewannouncement(response.POST)
if form.is_valid():
t = form.cleaned_data["text"]
a = Announcements(text=t)
a.save()
if form.cleaned_data["check"] == True:
send_message(t)
messages.success(
response, 'Announcement was saved and messages are sent')
else:
messages.success(response, 'Announcement was saved')
return render(response, "Teacher/home.html", {})
else:
form = createnewannouncement()
return render(response, "Teacher/announcement.html", {"form": form})
@allowed_users(allowed_roles=['admin', 'Professor'])
def create_class(response):
if response.method == "POST":
form = schedule_extra_class(response.POST)
if form.is_valid():
c1 = form.cleaned_data["subject"]
c2 = form.cleaned_data["date"]
c3 = form.cleaned_data["time_start"]
c4 = form.cleaned_data["time_end"]
c = Class(subject=c1, date=c2, time_start=c3, time_end=c4)
c.save()
t = "You have a {0} extra class on {1} from {2} to {3}".format(
str(c1), str(c2), str(c3), str(c4))
if form.cleaned_data["check"] == True:
send_message(t)
messages.success(
response, 'Class is scheduled and messages are sent')
else:
messages.success(response, 'Class is scheduled')
return render(response, "Teacher/home.html", {"text": t})
else:
form = schedule_extra_class()
return render(response, "Teacher/class.html", {"form": form})
@allowed_users(allowed_roles=['admin', 'Professor'])
def create_exam(response):
if response.method == "POST":
form = schedule_exam(response.POST)
if form.is_valid():
e1 = form.cleaned_data["subject"]
e2 = form.cleaned_data["date"]
e3 = form.cleaned_data["time_start"]
e4 = form.cleaned_data["time_end"]
e = Exam(subject=e1, date=e2, time_start=e3, time_end=e4)
e.save()
t = "You have a {0} exam on {1} from {2} to {3}".format(
str(e1), str(e2), str(e3), str(e4))
if form.cleaned_data["check"] == True:
send_message(t)
messages.success(
response, 'Exam is scheduled and messages are sent')
else:
messages.success(response, 'Exam is scheduled')
return render(response, "Teacher/home.html", {"text": t})
else:
form = schedule_exam()
return render(response, "Teacher/exam.html", {"form": form})
网址.py
此文件将来自不同依赖项和其他应用程序的 URL 链接到主项目。
蟒蛇3
from django.contrib import admin
from django.urls import path, include
from Register import views as v
urlpatterns = [
path('admin/', admin.site.urls),
path('', include("Teacher.urls")),
path('', include("Student.urls")),
path('register/', v.register, name="register"),
path("", include("django.contrib.auth.urls")),
]
现在让我们创建项目所需的应用程序。
注册应用
这个应用程序处理用户的登录和注册。
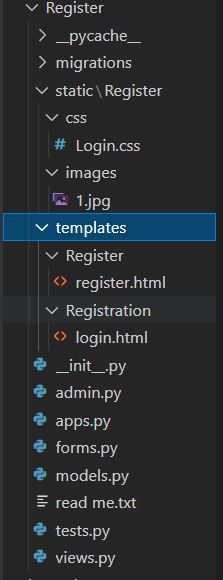
寄存器层次结构
注意: Static 文件夹包含所有静态文件,如 JavaScript 文件、CSS 文件和图像
模板文件夹
您可以看到有一个包含两个 HTML 文件的模板文件夹。第一个文件是 register.html,第二个文件是 login.html
HTML
{%load static%}
{% load crispy_forms_tags %}
Login/SignUp
{{form.errors}}
HTML
{%load static%}
{% if user.is_authenticated %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
You are already logged in
Click here if you are a professor
Click here if you are a student
{% else %}
{% load crispy_forms_tags %}
Login/SignUp
{{form.errors}}
Dont have an account? create one here
{% endif %}
应用程序.py
这将注册应用程序,因为它将使用数据库。
蟒蛇3
from django.apps import AppConfig
class RegisterConfig(AppConfig):
default_auto_field = 'django.db.models.BigAutoField'
name = 'Register'
表格.py
这里创建了一个自定义注册表单。
蟒蛇3
from django import forms
from django.contrib.auth import login, authenticate
from django.contrib.auth.forms import UserCreationForm
from django.contrib.auth.models import User
class RegistrationForm(UserCreationForm):
email = forms.EmailField()
class Meta:
model = User
# order in which the fields show up
fields = ["email", "username", "password1", "password2"]
视图.py
这个文件让我们可以管理页面的显示方式以及谁可以看到页面。
蟒蛇3
from django.shortcuts import render,redirect
from django.contrib.auth import login,authenticate,logout
from .forms import RegistrationForm
# Create your views here.
def register(request):
if request.user.is_authenticated:
return redirect("/")
if request.method == 'POST':
form=RegistrationForm(request.POST)
if form.is_valid():
form.save()
return redirect('login')
else:
form=RegistrationForm()
return render(request,"Register/register.html",{"form":form})
学生应用
这个应用程序处理项目的学生方面。
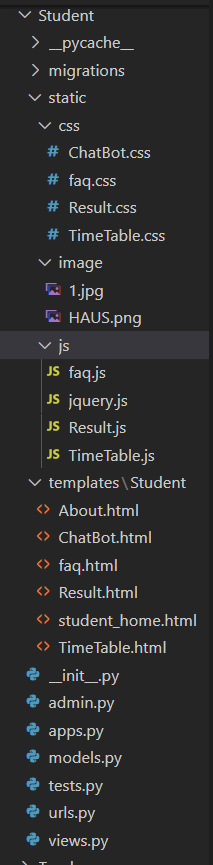
学生等级
模板:
About.html、ChatBot.html、faq.html、student_home.html 和 TimeTable.html
未登录的任何人都看不到这些页面。
HTML
{% load static %}
{% if user.is_authenticated %}
About Us
HAUS Developers
- Hardeep
- Ahmed
- Utsav
- Sarthak
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
ChatBot
ChatBot
Hi I am Era
Under Construction
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
FAQ
Frequently Asked Questions
Why shouldn't we trust atoms?
They make up everything
What do you call someone with no body and no nose?
Nobody knows.
What's the object-oriented way to become wealthy?
Inheritance.
How many tickles does it take to tickle an octopus?
Ten-tickles!
What is: 1 + 1?
Depends on who are you asking.
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
Student's Portal
HAUS-Connect
Student's Portal
HAUS-Connect is platform where all the faculties can send notice,Schedule Exam and Schedule Lectures. Any action performed by faculty will send a text message to all the students informing them about any upcoming events. HAUS-Connect aims to decrease the ridge between Student and Faculty.It is a firm of the Students,by the Students, for the Students.
Announcement
{% for t in t1 %}
{{ t }}
{% endfor %}
Lectures
{% for t in t2 %}
You have a {{t.subject}}
extra class on {{t.date}}
from {{t.time_start}}
to {{t.time_end}}
{% endfor %}
Exam
{% for t in t3 %}
You have a {{t.subject}}
extra class on {{t.date}}
from {{t.time_start}}
to {{t.time_end}}
{% endfor %}
© 2021 HAUS - All Rights Reserved
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
TIMETABLE
Sunday
Monday
Tuesday
Wednesday
Thursday
Friday
Saturday
8:00 AM - 9:00 AM
--
Data Structures
Digital Electronics
Microprocessor Programming
OOPS With Java
Open Elective
--
9:00 AM - 10:00 AM
--
Discrete Math
Discrete Math
OOPS With Java
Data Structures
Data Structures - Lab
--
10:00 AM - 11:00 AM
--
Open Elective
Data Structures - Lab
Data Structures
Discrete Math
OOPS With Java
--
11:00 AM - 12:00 PM
--
Microprocessor Programming - Lab
OOPS With Java
Open Elective
Digital Electronics - Lab
Microprocessor Programming
--
12:00 PM - 1:00 PM
--
Digital Electronics
Microprocessor Programming
Discrete Math - Tutorial
Microprocessor Programming - Lab
OOPS With Java - Lab
--
1:00 PM - 2:00 PM
--
Discrete Math
OOPS With Java - Lab
Data Structures
Digital Electronics
Discrete Math
--
2:00 PM - 3:00 PM
--
--
--
--
--
--
--
3:00 PM - 4:00 PM
--
--
--
--
--
--
--
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
视图.py
这个文件让我们可以管理页面的显示方式以及谁可以看到页面。
蟒蛇3
from Teacher.models import Announcements, Exam, Class
from django.shortcuts import render
from django.contrib.auth import logout
from django.http import HttpResponse, HttpResponseRedirect
# Create your views here.
def home(response):
t1 = Announcements.objects.all()
t2 = Class.objects.all()
t3 = Exam.objects.all()
return render(response, "Student/student_home.html",
{"t1": t1, "t2": t2, "t3": t3})
def chatbot(response):
return render(response, "Student/ChatBot.html")
def timetable(response):
return render(response, "Student/TimeTable.html")
def result(response):
return render(response, "Student/Result.html")
def faq(response):
return render(response, "Student/faq.html")
def logout_request(request):
logout(request)
return render(request, "Student/student_home.html", {})
def about(request):
return render(request, "Student/About.html", {})
网址.py
在这里,我们为模板设置路径。
蟒蛇3
from django.urls import path
from . import views
urlpatterns = [
path("student_home/", views.home, name="Home"),
path("chatbot/", views.chatbot, name="ChatBot"),
path("FAQ/", views.faq, name="FAQ"),
path("Result/", views.result, name="Result"),
path("TimeTable/", views.timetable, name="TimeTable"),
path("logout/", views.logout_request, name="logout"),
path("about/", views.about, name="About")
]
教师应用
这个应用程序处理项目的教师方面。
模板:
未登录的任何人都无法看到所有这些页面,并且不允许具有“学生”身份的帐户使用它们。
HTML
{% load static %}
{% if user.is_authenticated %}
Create Announcement
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
You dont have authorization to access this page.
If you are a professor contact administrator.
Click here if you are a student
HTML
{% load static %}
{% if user.is_authenticated %}
Schedule Class
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
Schedule Exam
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
{% load static %}
{% if user.is_authenticated %}
Teacher's Portal
{% include 'Teacher/messages.html' %}
HAUS-Connect
Teacher's Portal
Basically, here all Faculty's can send notice , Schedule Exam and Schedule Lectures. Any action performed by Faculty will send a text message to all students informing them about any upcoming events. HAUS-Connect aims to decrease the ridge between Student and Faculty.It is a firm of the Students,by the Students, for the Students.
TimeTable
*Note this is fixed timetable provided by the institution
We the students of PDEU CE`20 batch, appreciate you for putting all your efforts and helping us throughout the day from solving our 'silly' doubts to help us in any problem which we faced during college.
-Thanking You.
© 2021 HAUS - All Rights Reserved
{% else %}
{% block title %}Please Login {% endblock %}
HAUS
~
Connect
Sadly you are not logged in
Login Here
{% endif %}
HTML
Login here
{% load crispy_forms_tags %}
Register Here
HTML
{% if messages %}
{% for message in messages %}
{% endfor %}
{% endif %}
管理文件
这用于注册将使用数据库的模型
蟒蛇3
from django.contrib import admin
from .models import *
# Register your models here.
admin.site.register(Announcements)
admin.site.register(Class)
admin.site.register(Exam)
应用程序.py
注册应用程序
蟒蛇3
from django.apps import AppConfig
class TeacherConfig(AppConfig):
default_auto_field = 'django.db.models.BigAutoField'
name = 'Teacher'
装饰器.py
该文件使我们能够限制对页面的访问。
蟒蛇3
from django.http import HttpResponse
from django.shortcuts import redirect, render
def allowed_users(allowed_roles=[]):
def decorator(view_func):
def wrapper_func(request, *args, **kwargs):
group = None
if request.user.groups.exists():
group = request.user.groups.all()[0].name
if group in allowed_roles:
return view_func(request, *args, **kwargs)
else:
return render(request, "Teacher/base.html", {})
return wrapper_func
return decorator'
表格.py
创建表格
蟒蛇3
from django import forms
class createnewannouncement(forms.Form):
text = forms.CharField(label="Announcement", max_length=1000)
check = forms.BooleanField(label="Send message", required=False)
class schedule_extra_class(forms.Form):
subject = forms.CharField(label="Subject", max_length=20)
date = forms.CharField(label="Date", max_length=20)
time_start = forms.CharField(label="Starting Time", max_length=20)
time_end = forms.CharField(label="Ending Time", max_length=20)
check = forms.BooleanField(label="Send message", required=False)
class schedule_exam(forms.Form):
subject = forms.CharField(label="Subject ", max_length=20)
date = forms.CharField(label="Date", max_length=20)
time_start = forms.CharField(label="Starting Time", max_length=20)
time_end = forms.CharField(label="Ending Time", max_length=20)
check = forms.BooleanField(label="Send message", required=False)
模型.py
这会为表单设置数据库以将数据保存在
蟒蛇3
from django.db import models
class Announcements(models.Model):
text = models.TextField(max_length=500)
def __str__(self):
return self.text
class Class(models.Model):
subject = models.TextField(max_length=50)
date = models.TextField(max_length=50)
time_start = models.TextField(max_length=5)
time_end = models.TextField(max_length=5)
message = "You have a {0} extra class on {1} from {2} to {3}".format(
str(subject), str(date), str(time_start), str(time_end))
def __str__(self):
message = "You have a {0} extra class on {1} from {2} to {3}".format(
str(self.subject), str(self.date), str(self.time_start), str(self.time_end))
return self.message
class Exam(models.Model):
subject = models.TextField(max_length=50)
date = models.TextField(max_length=50)
time_start = models.TextField(max_length=5)
time_end = models.TextField(max_length=5)
message = "You have a {0} exam on {1} from {2} to {3}".format(
str(subject), str(date), str(time_start), str(time_end))
def __str__(self):
message = "You have a {0} exam on {1} from {2} to {3}".format(
str(self.subject), str(self.date), str(self.time_start), str(self.time_end))
return self.message
发送消息.py
此文件负责向提供的号码发送消息
蟒蛇3
def send_message(message):
from twilio.rest import Client
client = Client("Account SId", "Auth token")
numbers = ["+916351816925", "+91xxxxxxxxxx",
"+91xxxxxxxxxx", "+91xxxxxxxxxx"]
names = ["Sarthak", "Hardeep", "Utsav", "Ahmed"]
for i in range(4):
m = ("Hello {} ".format(names[i]))+message
client.messages.create(to=numbers[i],
from_="number provided by the api",
body=(m))
网址.py
将模板链接到地址
蟒蛇3
from django.urls import path
from . import views
urlpatterns = [
path("", views.home, name="Home"),
path("", views.index, name="index"),
path("announcement/", views.create_announcement, name="Announcement"),
path("class/", views.create_class, name="Extra Class"),
path("exam/", views.create_exam, name="Exams"),
path("logout/", views.logout_request, name="logout"),
path("base/", views.base, name="Base"),
]
视图.py
这个文件让我们可以管理页面的显示方式以及谁可以看到页面。这也是我们使用decorator.py 来限制访问的地方。
蟒蛇3
from datetime import date
from django.shortcuts import render
from django.contrib.auth import logout
from django.http import HttpResponse, HttpResponseRedirect
from django.contrib import messages
from .decorator import allowed_users
from .models import *
from .forms import *
from .Sending_messages import *
def base(request):
return render(request, "Teacher/base.html")
@allowed_users(allowed_roles=['admin', 'Professor'])
def home(response):
return render(response, "Teacher/home.html")
def logout_request(request):
logout(request)
return render(request, "Teacher/home.html", {})
@allowed_users(allowed_roles=['admin', 'Professor'])
def index(response, id):
a = Announcements.objects.get(id=id)
return render(response, "Teacher/home.html", {})
@allowed_users(allowed_roles=['admin', 'Professor'])
def create_announcement(response):
if response.method == "POST":
form = createnewannouncement(response.POST)
if form.is_valid():
t = form.cleaned_data["text"]
a = Announcements(text=t)
a.save()
if form.cleaned_data["check"] == True:
send_message(t)
messages.success(
response, 'Announcement was saved and messages are sent')
else:
messages.success(response, 'Announcement was saved')
return render(response, "Teacher/home.html", {})
else:
form = createnewannouncement()
return render(response, "Teacher/announcement.html", {"form": form})
@allowed_users(allowed_roles=['admin', 'Professor'])
def create_class(response):
if response.method == "POST":
form = schedule_extra_class(response.POST)
if form.is_valid():
c1 = form.cleaned_data["subject"]
c2 = form.cleaned_data["date"]
c3 = form.cleaned_data["time_start"]
c4 = form.cleaned_data["time_end"]
c = Class(subject=c1, date=c2, time_start=c3, time_end=c4)
c.save()
t = "You have a {0} extra class on {1} from {2} to {3}".format(
str(c1), str(c2), str(c3), str(c4))
if form.cleaned_data["check"] == True:
send_message(t)
messages.success(
response, 'Class is scheduled and messages are sent')
else:
messages.success(response, 'Class is scheduled')
return render(response, "Teacher/home.html", {"text": t})
else:
form = schedule_extra_class()
return render(response, "Teacher/class.html", {"form": form})
@allowed_users(allowed_roles=['admin', 'Professor'])
def create_exam(response):
if response.method == "POST":
form = schedule_exam(response.POST)
if form.is_valid():
e1 = form.cleaned_data["subject"]
e2 = form.cleaned_data["date"]
e3 = form.cleaned_data["time_start"]
e4 = form.cleaned_data["time_end"]
e = Exam(subject=e1, date=e2, time_start=e3, time_end=e4)
e.save()
t = "You have a {0} exam on {1} from {2} to {3}".format(
str(e1), str(e2), str(e3), str(e4))
if form.cleaned_data["check"] == True:
send_message(t)
messages.success(
response, 'Exam is scheduled and messages are sent')
else:
messages.success(response, 'Exam is scheduled')
return render(response, "Teacher/home.html", {"text": t})
else:
form = schedule_exam()
return render(response, "Teacher/exam.html", {"form": form})
输出
登录页面:
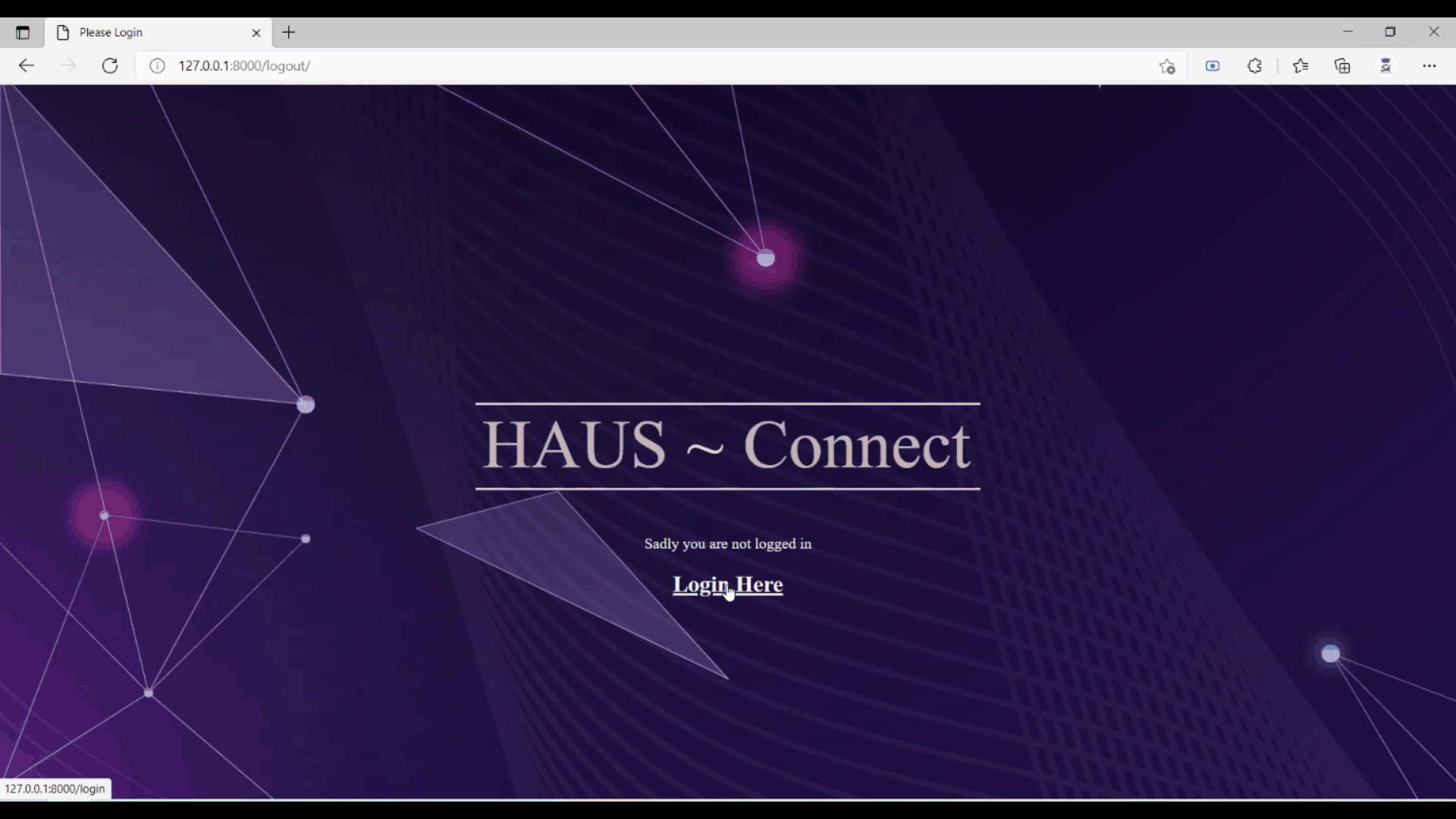
登录页面
来自教师门户的见解:

教师门户 – 主页

教师门户-通知/公告选项卡
还有其他选项卡,如考试和课程,教师可以在其中安排考试和课程。
来自学生门户的见解:

学生门户-首页
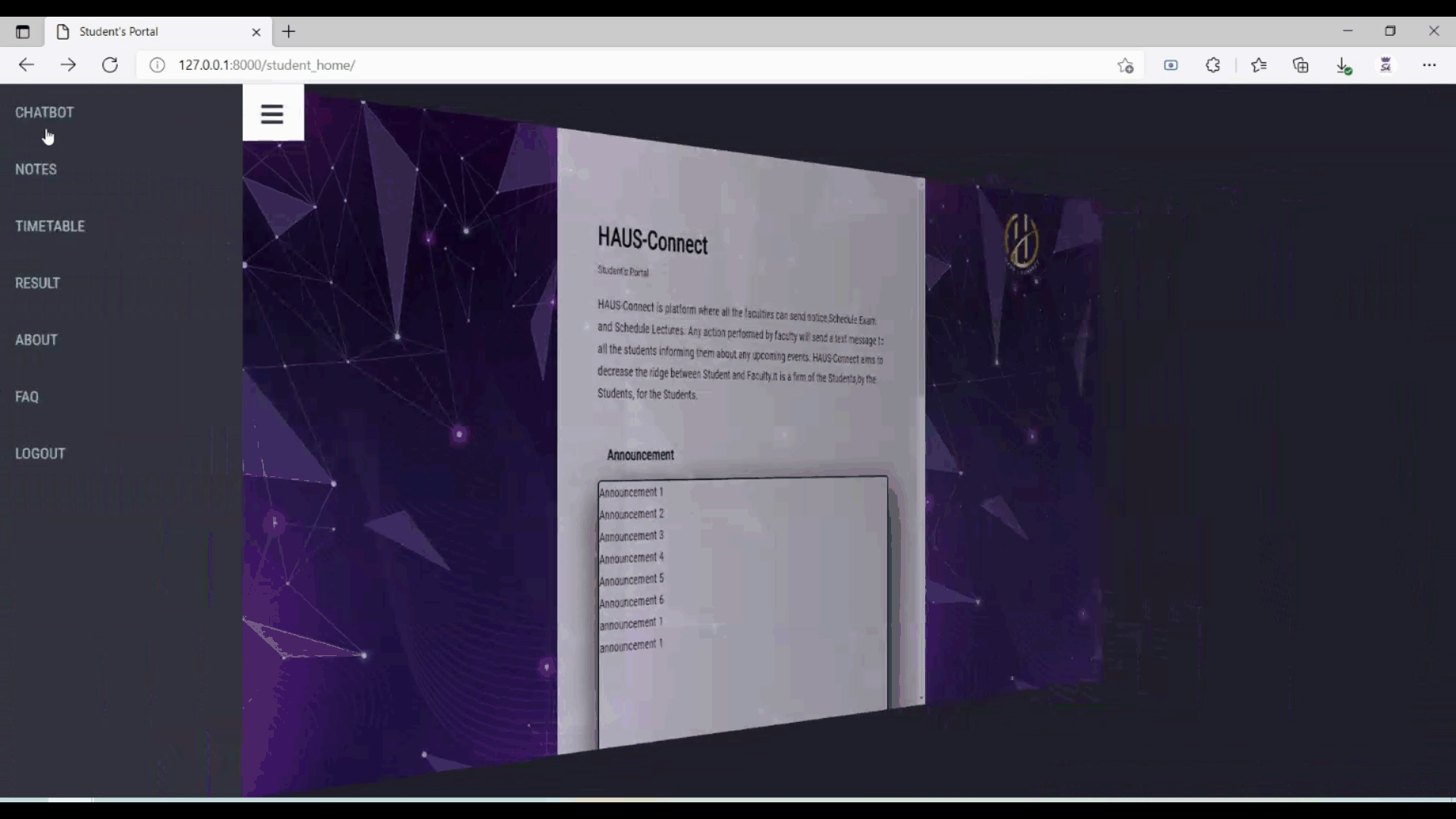
学生门户-时间表选项卡
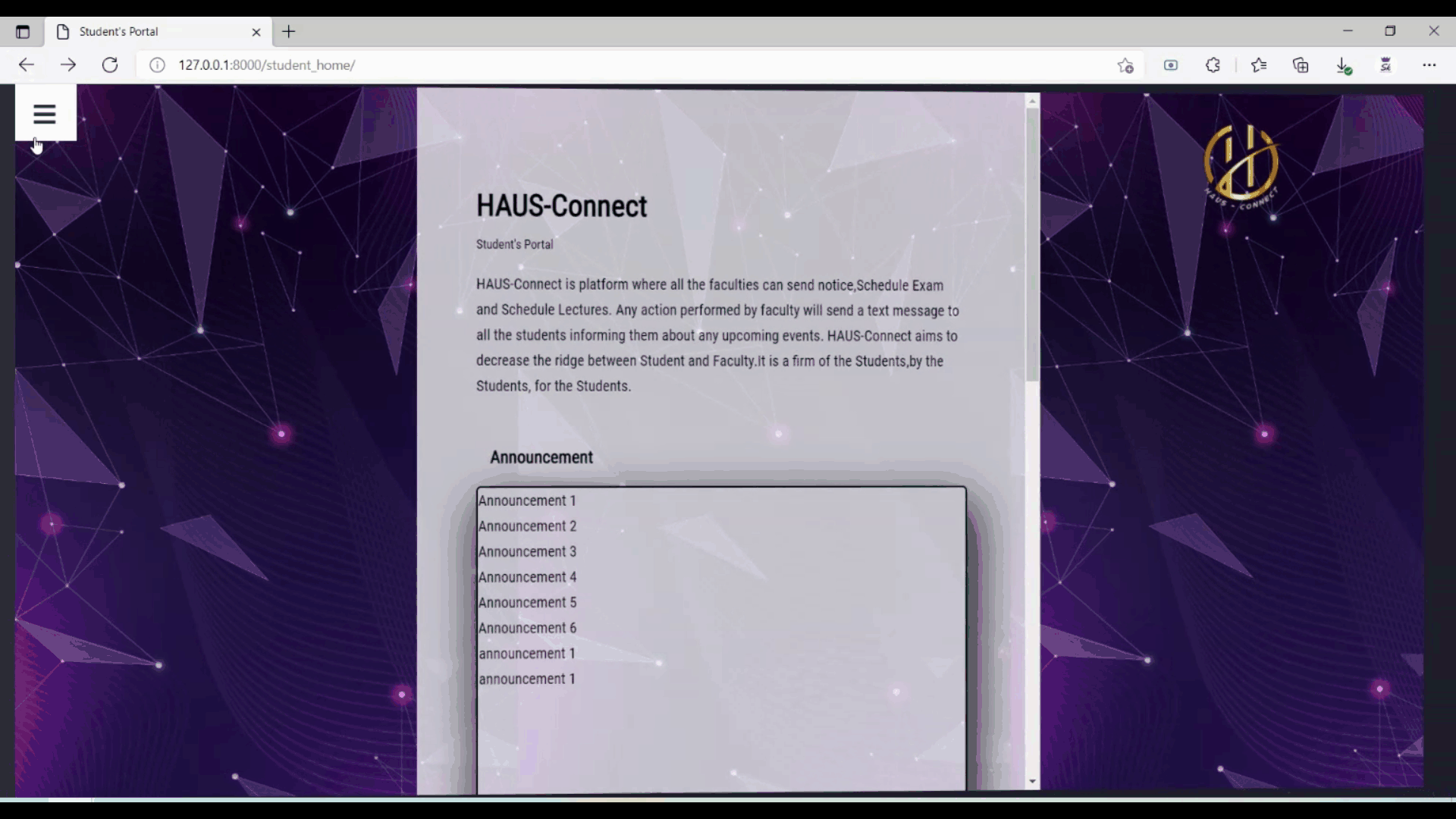
学生门户 - 结果选项卡

学生门户 – 关于我们和常见问题选项卡
还有其他选项卡,如聊天机器人和笔记。
注销选项:
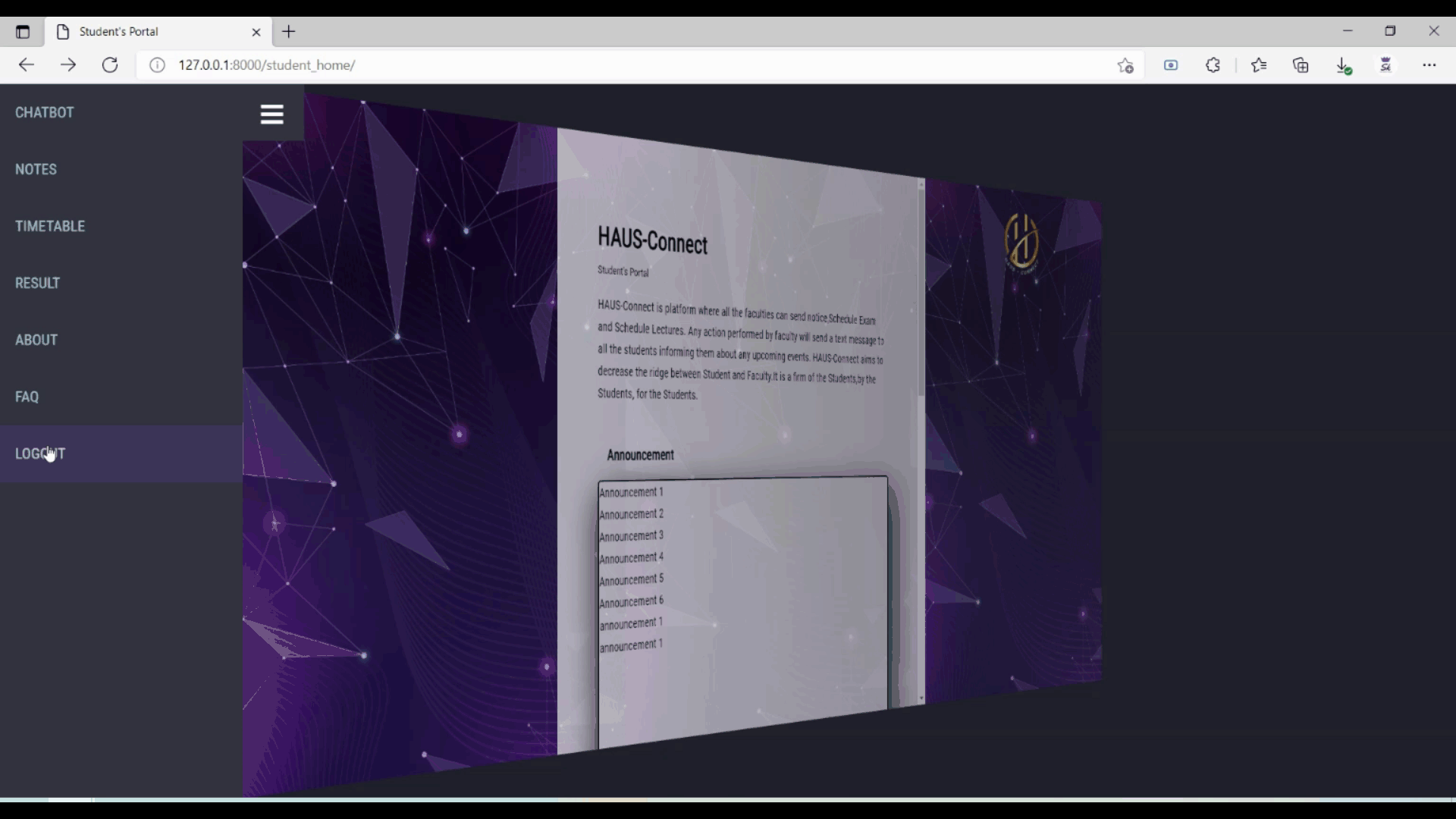
登出页面
GitHub 链接 – HAUS-Connect
团队成员
- 哈迪普·帕特尔
- 艾哈迈德·穆拉
- 乌察夫·梅塔
- 萨塔克·卡帕利亚