角度材质树
Angular Material是由 Google 开发的 UI 组件库,以便 Angular 开发人员可以以结构化和响应式的方式开发现代应用程序。通过使用这个库,我们可以极大地提高最终用户的用户体验,从而为我们的应用程序赢得人气。该库包含现代即用型元素,可以直接使用最少或无需额外代码。
- 平树
- 嵌套树
安装语法:
基本的先决条件是我们必须在系统上安装 Angular CLI 才能添加和配置 Angular 材质库。满足所需条件后,我们可以在 Angular CLI 上键入以下命令:
ng add @angular/material
有关详细的安装过程,请参阅将 Angular 材质组件添加到 Angular 应用程序一文。
添加 Mat-Tree 组件:
- 要使用 Flat Tree 组件,我们需要将其导入到我们的组件文件中,如下所示:
import { FlatTreeControl } from '@angular/cdk/tree';
- 要使用嵌套树组件,我们需要将其导入到我们的组件文件中,如下:
import { NestedTreeControl } from '@angular/cdk/tree';
- 为了使用Material Tree 模块,我们需要将其导入app.module.ts文件:
import {MatTreeModule} from '@angular/material/tree';
使用 import 语句后,将MatTreeModule也添加到 NgModule 导入数组中。
扁平树:扁平树是一棵树,其中所有节点都以顺序方式呈现在 DOM 上。可以将其视为以树格式一个接一个地显示数组元素。结果树具有可以展开/折叠的节点,并且 DOM 成为单深度列表。
句法:
Parent node name
Child Node 1
Child Node 2
项目结构:安装成功后,项目结构如下图所示:
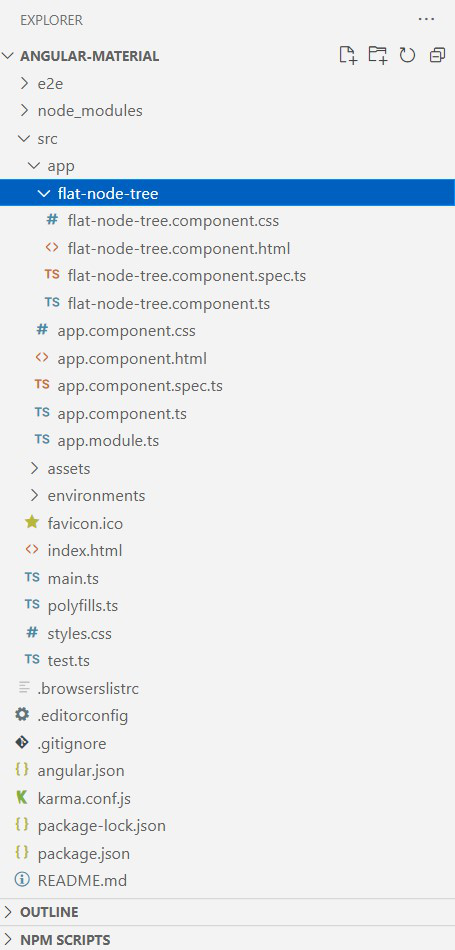
Flat Tree 的项目结构
示例:以下示例说明了 Angular Material Flat Tree 的实现。
flat-node-tree.component.ts
import { Component, OnInit } from "@angular/core";
import { FlatTreeControl } from "@angular/cdk/tree";
import { MatTreeFlatDataSource, MatTreeFlattener }
from "@angular/material/tree";
interface Family {
name: string;
children?: Family[];
}
const FAMILY_TREE: Family[] = [
{
name: "Joyce",
children: [
{ name: "Mike" },
{ name: "Will" },
{ name: "Eleven", children: [{ name: "Hopper" }] },
{ name: "Lucas" },
{ name: "Dustin", children: [{ name: "Winona" }] },
],
},
{
name: "Jean",
children: [{ name: "Otis" }, { name: "Maeve" }],
},
];
/** Flat node with expandable and level information */
interface ExampleFlatNode {
expandable: boolean;
name: string;
level: number;
}
@Component({
selector: "app-flat-node-tree",
templateUrl: "./flat-node-tree.component.html",
styleUrls: ["./flat-node-tree.component.css"],
})
export class FlatNodeTreeComponent implements OnInit {
private _transformer = (node: Family, level: number) => {
return {
expandable: !!node.children && node.children.length > 0,
name: node.name,
level: level,
};
};
treeControl = new FlatTreeControl(
(node) => node.level,
(node) => node.expandable
);
treeFlattener = new MatTreeFlattener(
this._transformer,
(node) => node.level,
(node) => node.expandable,
(node) => node.children
);
dataSource = new MatTreeFlatDataSource(
this.treeControl, this.treeFlattener);
constructor() {
this.dataSource.data = FAMILY_TREE;
}
hasChild = (_: number,
node: ExampleFlatNode) => node.expandable;
ngOnInit(): void {}
}
flat-node-tree.component.html
GeeksforGeeks
MatTree Example
{{node.name}}
{{node.name}}
flat-node-tree.component.css
h1, h3 {
color: green;
font-family: "Roboto", sans-serif;
text-align: center;
}
.mat-tree {
background: transparent;
}
.mat-tree-node {
color: black;
}
app.component.html
app.module.ts
import { CommonModule } from "@angular/common";
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations";
import { FlatNodeTreeComponent }
from "./flat-node-tree/flat-node-tree.component";
import { MatTreeModule } from "@angular/material/tree";
import { MatIconModule } from "@angular/material/icon";
@NgModule({
declarations: [AppComponent, FlatNodeTreeComponent],
exports: [AppComponent],
imports: [
CommonModule,
BrowserAnimationsModule,
BrowserModule,
MatTreeModule,
MatIconModule,
],
bootstrap: [AppComponent],
})
export class AppModule {}
nested-tree-example.component.ts
import { Component, OnInit } from "@angular/core";
import { NestedTreeControl } from "@angular/cdk/tree";
import { MatTreeNestedDataSource } from "@angular/material/tree";
interface Smartphone {
parent_company: string;
sub_brand?: Smartphone[];
}
const TREE_DATA: Smartphone[] = [
{
parent_company: "Xiaomi",
sub_brand: [
{ parent_company: "Poco" },
{ parent_company: "Redmi" },
{ parent_company: "Mijia" },
],
},
{
parent_company: "BBK Electronics",
sub_brand: [
{
parent_company: "Vivo",
sub_brand: [{ parent_company: "iQoo" }],
},
{
parent_company: "Oppo",
sub_brand: [{ parent_company: "Realme" },
{ parent_company: "Dizo" }],
},
],
},
];
@Component({
selector: "app-nested-tree-example",
templateUrl: "./nested-tree-example.component.html",
styleUrls: ["./nested-tree-example.component.css"],
})
export class NestedTreeExampleComponent implements OnInit {
treeControl = new NestedTreeControl((node) => node.sub_brand);
dataSource = new MatTreeNestedDataSource();
constructor() {
this.dataSource.data = TREE_DATA;
}
hasChild = (_: number, node: Smartphone) =>
!!node.sub_brand && node.sub_brand.length > 0;
ngOnInit(): void {}
}
nested-tree-example.component.html
GeeksforGeeks
Nested Tree Example
{{node.parent_company}}
{{node.parent_company}}
nested-tree-example.component.css
.example-tree-invisible {
display: none;
}
.example-tree ul,
.example-tree li {
margin-top: 0;
margin-bottom: 0;
list-style-type: none;
}
/* This padding sets alignment of the nested nodes. */
.example-tree .mat-nested-tree-node div[role="group"] {
padding-left: 40px;
}
.example-tree div[role="group"] > .mat-tree-node {
padding-left: 40px;
}
h1,
h3 {
color: green;
font-family: "Roboto", sans-serif;
text-align: left;
}
.mat-tree {
background: transparent;
}
.mat-tree-node {
color: black;
}
app.component.html
app.module.ts
import { CommonModule } from "@angular/common";
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations";
import { NestedTreeExampleComponent }
from "./nested-tree-example/nested-tree-example.component";
import { MatTreeModule } from "@angular/material/tree";
import { MatIconModule } from "@angular/material/icon";
@NgModule({
declarations: [AppComponent, NestedTreeExampleComponent],
exports: [AppComponent],
imports: [
CommonModule,
BrowserAnimationsModule,
BrowserModule,
MatTreeModule,
MatIconModule,
],
bootstrap: [AppComponent],
})
export class AppModule {}
平面节点树.component.html
GeeksforGeeks
MatTree Example
{{node.name}}
{{node.name}}
平面节点树.component.css
h1, h3 {
color: green;
font-family: "Roboto", sans-serif;
text-align: center;
}
.mat-tree {
background: transparent;
}
.mat-tree-node {
color: black;
}
app.component.html
app.module.ts
import { CommonModule } from "@angular/common";
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations";
import { FlatNodeTreeComponent }
from "./flat-node-tree/flat-node-tree.component";
import { MatTreeModule } from "@angular/material/tree";
import { MatIconModule } from "@angular/material/icon";
@NgModule({
declarations: [AppComponent, FlatNodeTreeComponent],
exports: [AppComponent],
imports: [
CommonModule,
BrowserAnimationsModule,
BrowserModule,
MatTreeModule,
MatIconModule,
],
bootstrap: [AppComponent],
})
export class AppModule {}
输出:
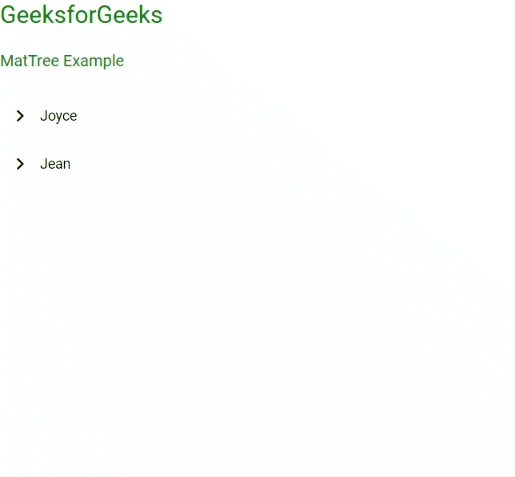
平面节点树示例
嵌套树:嵌套树,是一棵树,其中所有子节点都放置在 DOM 中的父节点内。当我们在节点之间有复杂的关系并且平面树无法创建所需的结构时,最好使用嵌套树。每个父节点都有自己的出口来定义子节点。
句法:
Parent node name
Child Node 1
Child Node 2
.
.
.
Child Node N
项目结构:安装成功后,项目结构如下图所示:
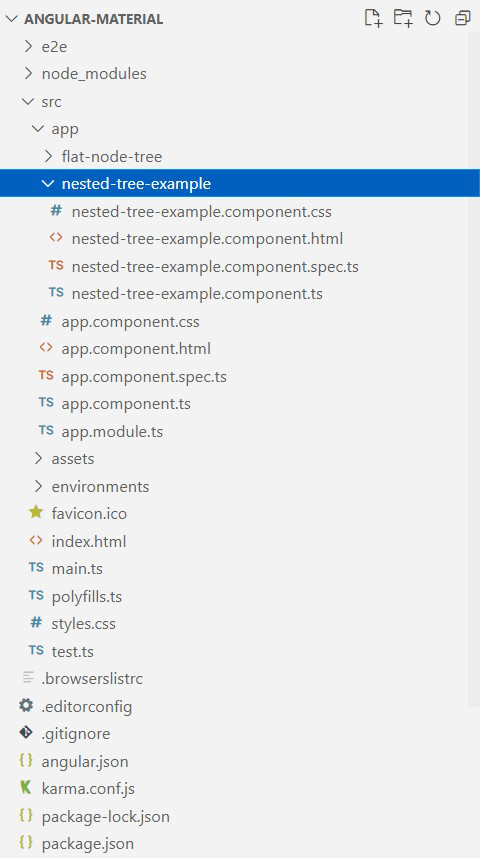
嵌套树的项目结构
示例:下面的示例说明了 Angular 材质嵌套树的实现。
嵌套树示例.component.ts
import { Component, OnInit } from "@angular/core";
import { NestedTreeControl } from "@angular/cdk/tree";
import { MatTreeNestedDataSource } from "@angular/material/tree";
interface Smartphone {
parent_company: string;
sub_brand?: Smartphone[];
}
const TREE_DATA: Smartphone[] = [
{
parent_company: "Xiaomi",
sub_brand: [
{ parent_company: "Poco" },
{ parent_company: "Redmi" },
{ parent_company: "Mijia" },
],
},
{
parent_company: "BBK Electronics",
sub_brand: [
{
parent_company: "Vivo",
sub_brand: [{ parent_company: "iQoo" }],
},
{
parent_company: "Oppo",
sub_brand: [{ parent_company: "Realme" },
{ parent_company: "Dizo" }],
},
],
},
];
@Component({
selector: "app-nested-tree-example",
templateUrl: "./nested-tree-example.component.html",
styleUrls: ["./nested-tree-example.component.css"],
})
export class NestedTreeExampleComponent implements OnInit {
treeControl = new NestedTreeControl((node) => node.sub_brand);
dataSource = new MatTreeNestedDataSource();
constructor() {
this.dataSource.data = TREE_DATA;
}
hasChild = (_: number, node: Smartphone) =>
!!node.sub_brand && node.sub_brand.length > 0;
ngOnInit(): void {}
}
嵌套树示例.component.html
GeeksforGeeks
Nested Tree Example
{{node.parent_company}}
{{node.parent_company}}
嵌套树示例.component.css
.example-tree-invisible {
display: none;
}
.example-tree ul,
.example-tree li {
margin-top: 0;
margin-bottom: 0;
list-style-type: none;
}
/* This padding sets alignment of the nested nodes. */
.example-tree .mat-nested-tree-node div[role="group"] {
padding-left: 40px;
}
.example-tree div[role="group"] > .mat-tree-node {
padding-left: 40px;
}
h1,
h3 {
color: green;
font-family: "Roboto", sans-serif;
text-align: left;
}
.mat-tree {
background: transparent;
}
.mat-tree-node {
color: black;
}
app.component.html
app.module.ts
import { CommonModule } from "@angular/common";
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations";
import { NestedTreeExampleComponent }
from "./nested-tree-example/nested-tree-example.component";
import { MatTreeModule } from "@angular/material/tree";
import { MatIconModule } from "@angular/material/icon";
@NgModule({
declarations: [AppComponent, NestedTreeExampleComponent],
exports: [AppComponent],
imports: [
CommonModule,
BrowserAnimationsModule,
BrowserModule,
MatTreeModule,
MatIconModule,
],
bootstrap: [AppComponent],
})
export class AppModule {}
输出:
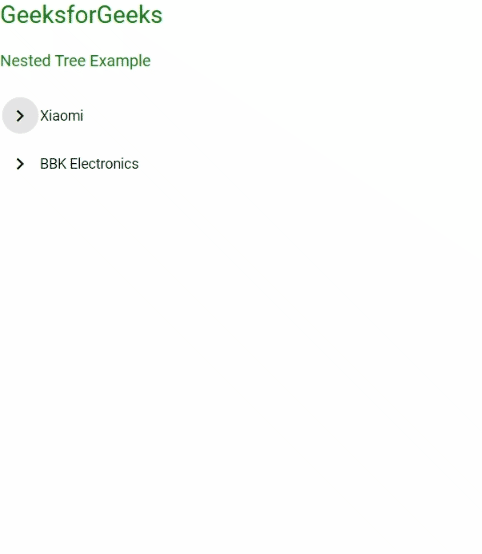
嵌套树示例
参考: https ://material.angular.io/components/tree/overview