Mongoose虚拟
虚拟是不持久或不存储在 MongoDB 数据库中的文档属性,它们仅在逻辑上存在并且不写入文档的集合。
通过虚拟属性的get方法,我们可以从已有的文档字段值中设置虚拟属性的值,并返回虚拟属性值。每次我们访问虚拟属性时, Mongoose都会调用 get 方法。
安装模块:
第 1 步:您可以访问链接 Install mongoose以获取有关安装mongoose模块的信息。您可以使用以下命令安装此软件包:
npm install mongoose
第 2 步:安装mongoose模块后,您可以使用以下代码将其导入到您的文件中:
const mongoose = require('mongoose');
数据库:最初,我们在数据库GFG中有一个空的用户集合。
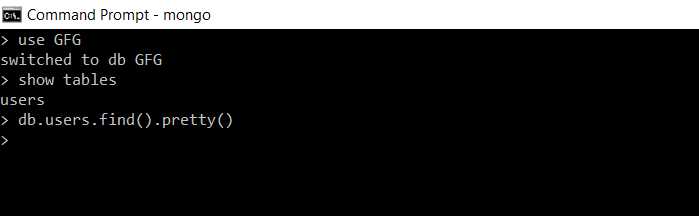
最初,集合用户为空
Get方法的实现:
文件名:app.js
Javascript
// Requiring module
const mongoose = require('mongoose');
const express = require('express');
const app = express();
// Connecting to database
mongoose.connect('mongodb://localhost:27017/GFG',
{
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false
});
// Constructing mongoose schema
const userSchema = new mongoose.Schema({
name: {
first: String,
last: String
}
});
// Setting virtual property using get method
userSchema.virtual('name.full')
.get(function () {
return this.name.first + ' ' + this.name.last;
})
// Creating mongoose model
const User = mongoose.model('user', userSchema);
const newUser = new User({
name: {
first: "David",
last: "Beckham"
}
})
newUser.save()
.then(u => {
console.log("USERNAME: ", u.name.full)
})
.catch(error => {
console.log(error);
})
Javascript
// Requiring module
const mongoose = require('mongoose');
const express = require('express');
const app = express();
// Connecting to database
mongoose.connect('mongodb://localhost:27017/GFG',
{
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false
});
// Constructing mongoose schema
const userSchema = new mongoose.Schema({
name: {
first: String,
last: String
}
});
// Setting the firstname and lastname using set method
userSchema.virtual('name.full')
.get(function () {
return this.name.first + ' ' + this.name.last;
})
.set(function (value) {
var fname = value.split(' ');
this.name.first = fname[0];
this.name.last = fname[1];
})
// Creating mongoose model
const User = mongoose.model('user', userSchema);
const newUser = new User({
name: {
full: "Dave Bautista"
}
})
newUser.save()
.then(u => {
console.log("FIRSTNAME: ", u.name.first,
"\nLASTNAME:", u.name.last)
})
.catch(error => {
console.log(error);
})
使用以下命令运行app.js文件:
node app.js
输出:
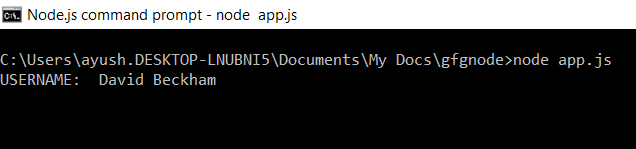
执行 app.js 后的输出
数据库:程序执行后,我们的数据库会是这个样子。
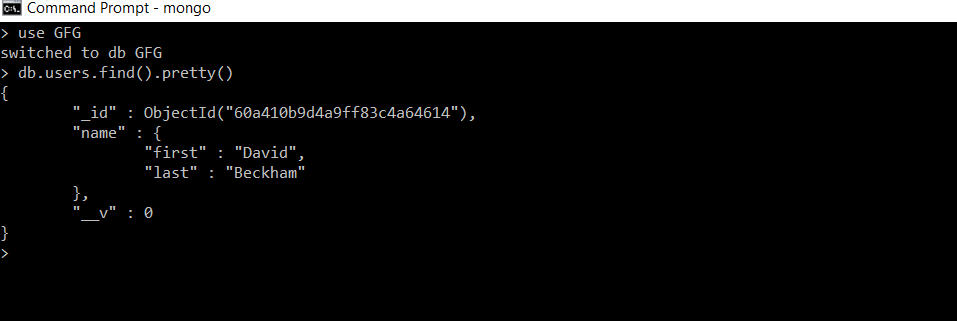
执行 app.js 后收集用户
说明:这里的模式包含字段name.first和name.last并且有一个虚拟属性name.full 。每当访问name.full时,都会调用 get 方法,我们将全名作为名字和姓氏的串联。因此,每当我们必须获取全名时,我们不必分别访问 firstname 和 lastname 并将它们连接起来,而是可以通过虚拟属性轻松获取它们。
使用虚拟属性的set方法,我们可以从虚拟属性的值中设置现有文档字段的值。
Set方法的实现:
文件名:index.js
Javascript
// Requiring module
const mongoose = require('mongoose');
const express = require('express');
const app = express();
// Connecting to database
mongoose.connect('mongodb://localhost:27017/GFG',
{
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false
});
// Constructing mongoose schema
const userSchema = new mongoose.Schema({
name: {
first: String,
last: String
}
});
// Setting the firstname and lastname using set method
userSchema.virtual('name.full')
.get(function () {
return this.name.first + ' ' + this.name.last;
})
.set(function (value) {
var fname = value.split(' ');
this.name.first = fname[0];
this.name.last = fname[1];
})
// Creating mongoose model
const User = mongoose.model('user', userSchema);
const newUser = new User({
name: {
full: "Dave Bautista"
}
})
newUser.save()
.then(u => {
console.log("FIRSTNAME: ", u.name.first,
"\nLASTNAME:", u.name.last)
})
.catch(error => {
console.log(error);
})
使用以下命令运行index.js文件:
node index.js
输出:
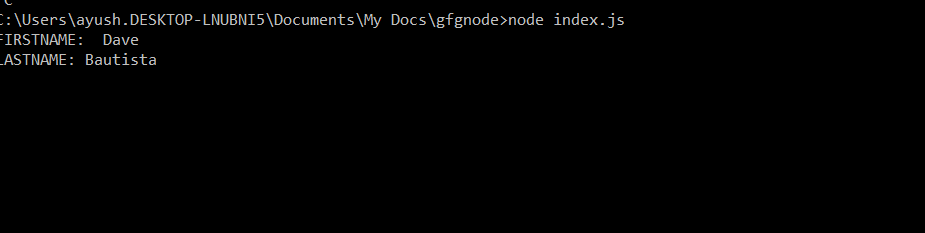
执行 index.js 后的输出
数据库:程序执行后,我们的数据库会是这个样子。
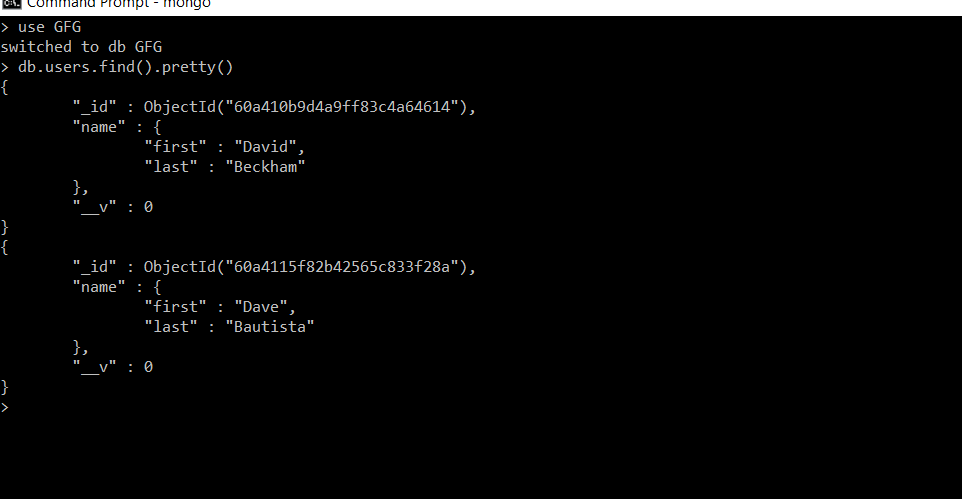
执行 index.js 后收集用户
说明:这里当用户使用虚拟属性name.full保存文档时,set函数会自动使用name.full设置字段name.first和name.last字段值。因此,在虚拟属性的帮助下,我们不需要单独提供每个字段来创建文档。