Java中的继承
继承是OOP(面向对象编程)的重要支柱。它是Java中允许一个类继承另一个类的特性(字段和方法)的机制。
重要术语:
- 超类:其特征被继承的类称为超类(或基类或父类)。
- 子类:继承另一个类的类称为子类(或派生类、扩展类或子类)。除了超类的字段和方法外,子类还可以添加自己的字段和方法。
- 可重用性:继承支持“可重用性”的概念,即当我们想要创建一个新类并且已经有一个类包含我们想要的一些代码时,我们可以从现有类中派生出我们的新类。通过这样做,我们正在重用现有类的字段和方法。
如何在Java中使用继承
用于继承的关键字是extends 。
句法 :
class derived-class extends base-class
{
//methods and fields
}
示例:在下面的继承示例中,Bicycle 类是基类,MountainBike 类是扩展 Bicycle 类的派生类,Test 类是运行程序的驱动程序类。
Java
// Java program to illustrate the
// concept of inheritance
// base class
class Bicycle {
// the Bicycle class has two fields
public int gear;
public int speed;
// the Bicycle class has one constructor
public Bicycle(int gear, int speed)
{
this.gear = gear;
this.speed = speed;
}
// the Bicycle class has three methods
public void applyBrake(int decrement)
{
speed -= decrement;
}
public void speedUp(int increment)
{
speed += increment;
}
// toString() method to print info of Bicycle
public String toString()
{
return ("No of gears are " + gear + "\n"
+ "speed of bicycle is " + speed);
}
}
// derived class
class MountainBike extends Bicycle {
// the MountainBike subclass adds one more field
public int seatHeight;
// the MountainBike subclass has one constructor
public MountainBike(int gear, int speed,
int startHeight)
{
// invoking base-class(Bicycle) constructor
super(gear, speed);
seatHeight = startHeight;
}
// the MountainBike subclass adds one more method
public void setHeight(int newValue)
{
seatHeight = newValue;
}
// overriding toString() method
// of Bicycle to print more info
@Override public String toString()
{
return (super.toString() + "\nseat height is "
+ seatHeight);
}
}
// driver class
public class Test {
public static void main(String args[])
{
MountainBike mb = new MountainBike(3, 100, 25);
System.out.println(mb.toString());
}
}
Java
// Java program to illustrate the
// concept of single inheritance
import java.io.*;
import java.lang.*;
import java.util.*;
class one {
public void print_geek()
{
System.out.println("Geeks");
}
}
class two extends one {
public void print_for() { System.out.println("for"); }
}
// Driver class
public class Main {
public static void main(String[] args)
{
two g = new two();
g.print_geek();
g.print_for();
g.print_geek();
}
}
Java
// Java program to illustrate the
// concept of Multilevel inheritance
import java.io.*;
import java.lang.*;
import java.util.*;
class one {
public void print_geek()
{
System.out.println("Geeks");
}
}
class two extends one {
public void print_for() { System.out.println("for"); }
}
class three extends two {
public void print_geek()
{
System.out.println("Geeks");
}
}
// Drived class
public class Main {
public static void main(String[] args)
{
three g = new three();
g.print_geek();
g.print_for();
g.print_geek();
}
}
Java
// Java program to illustrate the
// concept of Hierarchical inheritance
class A {
public void print_A() { System.out.println("Class A"); }
}
class B extends A {
public void print_B() { System.out.println("Class B"); }
}
class C extends A {
public void print_C() { System.out.println("Class C"); }
}
class D extends A {
public void print_D() { System.out.println("Class D"); }
}
// Driver Class
public class Test {
public static void main(String[] args)
{
B obj_B = new B();
obj_B.print_A();
obj_B.print_B();
C obj_C = new C();
obj_C.print_A();
obj_C.print_C();
D obj_D = new D();
obj_D.print_A();
obj_D.print_D();
}
}
Java
// Java program to illustrate the
// concept of Multiple inheritance
import java.io.*;
import java.lang.*;
import java.util.*;
interface one {
public void print_geek();
}
interface two {
public void print_for();
}
interface three extends one, two {
public void print_geek();
}
class child implements three {
@Override public void print_geek()
{
System.out.println("Geeks");
}
public void print_for() { System.out.println("for"); }
}
// Drived class
public class Main {
public static void main(String[] args)
{
child c = new child();
c.print_geek();
c.print_for();
c.print_geek();
}
}
Java
public class SolarSystem {
}
public class Earth extends SolarSystem {
}
public class Mars extends SolarSystem {
}
public class Moon extends Earth {
}
Java
class SolarSystem {
}
class Earth extends SolarSystem {
}
class Mars extends SolarSystem {
}
public class Moon extends Earth {
public static void main(String args[])
{
SolarSystem s = new SolarSystem();
Earth e = new Earth();
Mars m = new Mars();
System.out.println(s instanceof SolarSystem);
System.out.println(e instanceof Earth);
System.out.println(m instanceof SolarSystem);
}
}
No of gears are 3
speed of bicycle is 100
seat height is 25
在上面的程序中,当创建一个 MountainBike 类的对象时,超类的所有方法和字段的副本都会在该对象中获取内存。这就是为什么通过使用子类的对象,我们也可以访问超类的成员。
请注意,在继承期间只创建子类的对象,而不是超类。有关更多信息,请参阅继承类的Java对象创建。
该程序的说明性图像:
在实践中,继承和多态在Java中一起使用,以实现代码的快速性能和可读性。
Java中的继承类型
以下是Java支持的不同类型的继承。
1. 单一继承:在单一继承中,子类继承了一个超类的特性。在下图中,A 类作为派生类 B 的基类。
Java
// Java program to illustrate the
// concept of single inheritance
import java.io.*;
import java.lang.*;
import java.util.*;
class one {
public void print_geek()
{
System.out.println("Geeks");
}
}
class two extends one {
public void print_for() { System.out.println("for"); }
}
// Driver class
public class Main {
public static void main(String[] args)
{
two g = new two();
g.print_geek();
g.print_for();
g.print_geek();
}
}
Geeks
for
Geeks
2.多级继承:在多级继承中,派生类将继承一个基类,并且派生类也充当其他类的基类。在下图中,A 类作为派生类 B 的基类,而 B 又作为派生类 C 的基类。在Java中,类不能直接访问祖父母的成员。
Java
// Java program to illustrate the
// concept of Multilevel inheritance
import java.io.*;
import java.lang.*;
import java.util.*;
class one {
public void print_geek()
{
System.out.println("Geeks");
}
}
class two extends one {
public void print_for() { System.out.println("for"); }
}
class three extends two {
public void print_geek()
{
System.out.println("Geeks");
}
}
// Drived class
public class Main {
public static void main(String[] args)
{
three g = new three();
g.print_geek();
g.print_for();
g.print_geek();
}
}
Geeks
for
Geeks
3.分层继承:在分层继承中,一个类充当多个子类的超类(基类)。在下图中,A 类作为派生类 B、C 和 D 的基类。
Java
// Java program to illustrate the
// concept of Hierarchical inheritance
class A {
public void print_A() { System.out.println("Class A"); }
}
class B extends A {
public void print_B() { System.out.println("Class B"); }
}
class C extends A {
public void print_C() { System.out.println("Class C"); }
}
class D extends A {
public void print_D() { System.out.println("Class D"); }
}
// Driver Class
public class Test {
public static void main(String[] args)
{
B obj_B = new B();
obj_B.print_A();
obj_B.print_B();
C obj_C = new C();
obj_C.print_A();
obj_C.print_C();
D obj_D = new D();
obj_D.print_A();
obj_D.print_D();
}
}
Class A
Class B
Class A
Class C
Class A
Class D
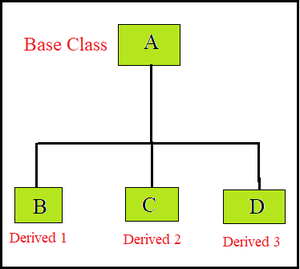
分层继承
4.多重继承(通过接口):在多重继承中,一个类可以有多个超类,并继承所有父类的特性。请注意, Java不支持类的多重继承。在Java中,我们只能通过Interfaces来实现多重继承。在下图中,C 类派生自接口 A 和 B。
Java
// Java program to illustrate the
// concept of Multiple inheritance
import java.io.*;
import java.lang.*;
import java.util.*;
interface one {
public void print_geek();
}
interface two {
public void print_for();
}
interface three extends one, two {
public void print_geek();
}
class child implements three {
@Override public void print_geek()
{
System.out.println("Geeks");
}
public void print_for() { System.out.println("for"); }
}
// Drived class
public class Main {
public static void main(String[] args)
{
child c = new child();
c.print_geek();
c.print_for();
c.print_geek();
}
}
Geeks
for
Geeks
5. Hybrid Inheritance(Through Interfaces):是上述两种或多种继承类型的混合。由于Java不支持类的多重继承,因此类也无法实现混合继承。在Java中,我们只能通过Interfaces来实现混合继承。
关于Java继承的重要事实
- 默认超类:除了没有超类的Object类,每个类都有一个且只有一个直接超类(单继承)。在没有任何其他显式超类的情况下,每个类都隐含地是 Object 类的子类。
- 超类只能是一个:超类可以有任意数量的子类。但是一个子类只能有一个超类。这是因为Java不支持类的多重继承。尽管使用接口,但Java支持多重继承。
- 继承构造函数:子类从其超类继承所有成员(字段、方法和嵌套类)。构造函数不是成员,所以子类不能继承,但是子类可以调用超类的构造函数。
- 私有成员继承:子类不继承其父类的私有成员。但是,如果超类具有用于访问其私有字段的公共或受保护方法(如 getter 和 setter),则子类也可以使用这些方法。
Java IS-一种关系。
IS-A 是一种说法:这个对象是那个对象的一个类型。让我们看看extends关键字是如何实现继承的。
Java
public class SolarSystem {
}
public class Earth extends SolarSystem {
}
public class Mars extends SolarSystem {
}
public class Moon extends Earth {
}
现在,基于上面的例子,在面向对象的术语中,以下是正确的:-
- SolarSystem 是 Earth 类的超类。
- SolarSystem 是 Mars 类的超类。
- Earth 和 Mars 是 SolarSystem 类的子类。
- Moon 是 Earth 和 SolarSystem 类的子类。
Java
class SolarSystem {
}
class Earth extends SolarSystem {
}
class Mars extends SolarSystem {
}
public class Moon extends Earth {
public static void main(String args[])
{
SolarSystem s = new SolarSystem();
Earth e = new Earth();
Mars m = new Mars();
System.out.println(s instanceof SolarSystem);
System.out.println(e instanceof Earth);
System.out.println(m instanceof SolarSystem);
}
}
true
true
true
在子类中可以做什么?
在子类中,我们可以按原样继承成员、替换它们、隐藏它们或用新成员补充它们:
- 继承的字段可以直接使用,就像任何其他字段一样。
- 我们可以在子类中声明不在超类中的新字段。
- 继承的方法可以直接使用。
- 我们可以在子类中编写一个与超类具有相同签名的新实例方法,从而覆盖它(如上例中, toString()方法被覆盖)。
- 我们可以在子类中编写一个与超类具有相同签名的新静态方法,从而隐藏它。
- 我们可以在子类中声明不在超类中的新方法。
- 我们可以编写一个子类构造函数来调用超类的构造函数,或者隐式调用,或者使用关键字 super。