Java程序打印数组中具有频率的所有重复数字
数组中某个元素的频率是该特定元素在整个数组中出现的次数。给定一个可能包含重复项的数组,打印所有重复/重复的元素及其频率。
下面是通过两种方式对这个程序的讨论:
- 使用计数器数组:通过维护一个单独的数组来维护每个元素的计数。
- 使用 HashMap :通过更新 HashMap
中每个数组元素的计数。
例子 :
Input : arr[] = {1, 2, 2, 1, 1, 2, 5, 2}
Output : 1 3
2 4
// here we will not print 5 as it is not repeated
Input : arr[] = {1, 2, 3}
Output : NULL
// output will be NULL as no element is repeated.
方法一:(使用计数器数组)
解释 :
- 数组可以排序也可以不排序。
- 首先,使用另一个数组计算数组中的所有数字。
- A 是一个数组, A[ ] = {1, 6 ,4 ,6, 4, 8, 2, 4, 1, 1}
- B 是一个计数器数组 B[x] = {0},其中 x = 数组 A 中的最大值“对于上面的示例 8”。
- 在 for 循环中,用 i 初始化。为数组 A 中的每个元素增加计数器数组中的值。
- B [ A [i] ]++;这将给计数器数组作为
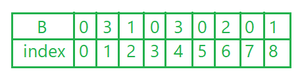
计算元素后的数组 B
- 最后,只要元素大于 1,就打印 B 及其元素的索引。
Java
// Java program to count the frequency of
// elements in an array by using an extra
// counter array of size equal to the maximum
// element of an array
import java.io.*;
class GFG {
public static void main(String[] args)
{
int A[] = { 1, 6, 4, 6, 4, 8, 2, 4, 1, 1 };
int max = Integer.MIN_VALUE;
for (int i = 0; i < A.length; i++) {
if (A[i] > max)
max = A[i];
}
int B[] = new int[max + 1];
for (int i = 0; i < A.length; i++) {
// increment in array B for every integer
// in A.
B[A[i]]++;
}
for (int i = 0; i <= max; i++) {
// output only if element is more than
// 1 time in array A.
if (B[i] > 1)
System.out.println(i + "-" + B[i]);
}
}
}
Java
// Java program to maintain
// the count of each element
// by using map.
import java.util.*;
class GFG {
public static void main(String[] args)
{
int[] a = { 1, 6, 4, 6, 4, 8, 2, 4, 1, 1 };
int n = a.length; // size of array
HashMap map = new HashMap<>();
for (int i = 0; i < n; i++) {
if (map.containsKey(a[i])) {
// if element is already in map
// then increase the value of element at
// index by 1
int c = map.get(a[i]);
map.replace(a[i], c + 1);
}
// if element is not in map than assign it by 1.
else
map.put(a[i], 1);
}
for (Map.Entry i :
map.entrySet()) {
// print only if count of element is greater
// than 1.
if (i.getValue() > 1)
System.out.println(i.getKey() + " "
+ i.getValue());
else
continue;
}
}
}
输出:
1-3
4-3
6-2
上面的方法可以消耗更多的空间,等于数组元素的最大约束
时间复杂度: O(n)
空间复杂度: O(K) 其中 K 是数组的最大元素/约束
方法二:(使用HashMap)
- 它是一种简单的方法并且具有基本逻辑,您也可以使用无序 Map 来实现。
- 该地图将节省大量空间和时间。
Java
// Java program to maintain
// the count of each element
// by using map.
import java.util.*;
class GFG {
public static void main(String[] args)
{
int[] a = { 1, 6, 4, 6, 4, 8, 2, 4, 1, 1 };
int n = a.length; // size of array
HashMap map = new HashMap<>();
for (int i = 0; i < n; i++) {
if (map.containsKey(a[i])) {
// if element is already in map
// then increase the value of element at
// index by 1
int c = map.get(a[i]);
map.replace(a[i], c + 1);
}
// if element is not in map than assign it by 1.
else
map.put(a[i], 1);
}
for (Map.Entry i :
map.entrySet()) {
// print only if count of element is greater
// than 1.
if (i.getValue() > 1)
System.out.println(i.getKey() + " "
+ i.getValue());
else
continue;
}
}
}
输出
1 3
4 3
6 2
时间复杂度: O(n)
空间复杂度: O(n)