给定两个圆,圆心为C1(x1, y1) 和 C2(x2, y2) ,半径为 r1 和 r2 ,任务是检查两个圆是否正交。
如果两条曲线的交角是直角,即它们的交点处的切线是垂直的,则称两条曲线是正交的。
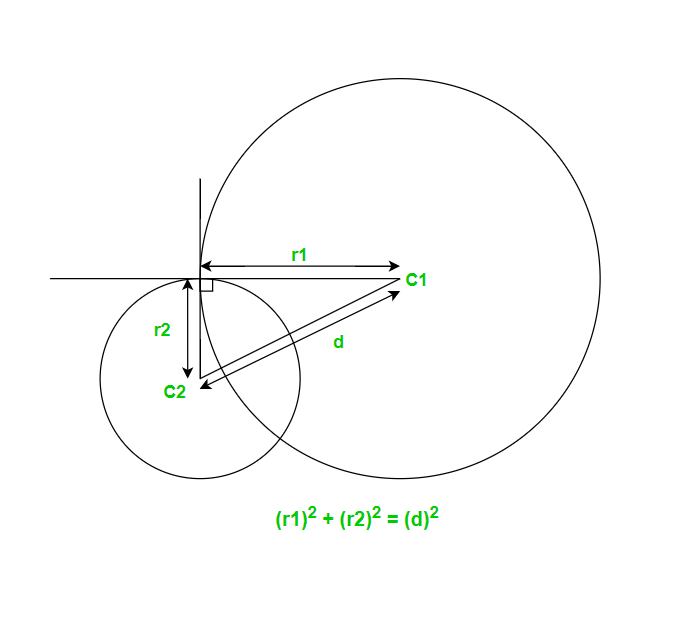
以上两个圆是正交的
例子:
Input: C1(4, 3), C2(0, 1), r1 = 2, r2 = 4
Output: Yes
Input: C1(4, 3), C2(1, 2), r1 = 2, r2 = 2
Output: No
方法:
- 用距离公式求两个圆“d”中心之间的距离。
- 为了使圆正交,我们需要检查是否
r1 * r1 + r2 * r2 = d * d
- 如果为真,则两个圆都是正交的。否则不行。
下面是上述方法的实现:
C++
// C++ program to check if two
// circles are orthogonal or not
#include
using namespace std;
// Function to Check if the given
// circles are orthogonal
bool orthogonality(int x1, int y1, int x2,
int y2, int r1, int r2)
{
// calculating the square
// of the distance between C1 and C2
int dsquare = (x1 - x2) * (x1 - x2)
+ (y1 - y2) * (y1 - y2);
// Check if the given
// circles are orthogonal
if (dsquare == r1 * r1 + r2 * r2)
return true;
else
return false;
}
// Driver code
int main()
{
int x1 = 4, y1 = 3;
int x2 = 0, y2 = 1;
int r1 = 2, r2 = 4;
bool f = orthogonality(x1, y1, x2,
y2, r1, r2);
if (f)
cout << "Given circles are"
<< " orthogonal.";
else
cout << "Given circles are"
<< " not orthogonal.";
return 0;
}
Java
// Java program to check if two
// circ
import java.util.*;
import java.lang.*;
import java.io.*;
class GFG
{
// Function to Check if the given
// circles are orthogonal
public static boolean orthogonality(int x1, int y1, int x2,
int y2, int r1, int r2)
{
// calculating the square
// of the distance between C1 and C2
int dsquare = (x1 - x2) * (x1 - x2) +
(y1 - y2) * (y1 - y2);
// Check if the given
// circles are orthogonal
if (dsquare == r1 * r1 + r2 * r2)
return true;
else
return false;
}
// Driver Code
public static void main(String[] args) throws java.lang.Exception
{
int x1 = 4, y1 = 3;
int x2 = 0, y2 = 1;
int r1 = 2, r2 = 4;
boolean f = orthogonality(x1, y1, x2, y2, r1, r2);
if (f)
System.out.println("Given circles are orthogonal.");
else
System.out.println("Given circles are not orthogonal.");
}
}
// This code is contributed by ashutosh450
Python3
# Python3 program to check if two
# circles are orthogonal or not
# Function to Check if the given
# circles are orthogonal
def orthogonality(x1, y1, x2, y2, r1, r2):
# calculating the square
# of the distance between C1 and C2
dsquare = (x1 - x2) * (x1 - x2) + \
(y1 - y2) * (y1 - y2);
# Check if the given
# circles are orthogonal
if (dsquare == r1 * r1 + r2 * r2):
return True
else:
return False
# Driver code
x1, y1 = 4, 3
x2, y2 = 0, 1
r1, r2 = 2, 4
f = orthogonality(x1, y1, x2, y2, r1, r2)
if (f):
print("Given circles are orthogonal.")
else:
print("Given circles are not orthogonal.")
# This code is contributed by Mohit Kumar
C#
// C# implementation for above program
using System;
class GFG
{
// Function to Check if the given
// circles are orthogonal
public static bool orthogonality(int x1, int y1, int x2,
int y2, int r1, int r2)
{
// calculating the square
// of the distance between C1 and C2
int dsquare = (x1 - x2) * (x1 - x2) +
(y1 - y2) * (y1 - y2);
// Check if the given
// circles are orthogonal
if (dsquare == r1 * r1 + r2 * r2)
return true;
else
return false;
}
// Driver Code
public static void Main()
{
int x1 = 4, y1 = 3;
int x2 = 0, y2 = 1;
int r1 = 2, r2 = 4;
bool f = orthogonality(x1, y1, x2, y2, r1, r2);
if (f)
Console.WriteLine("Given circles are orthogonal.");
else
Console.WriteLine("Given circles are not orthogonal.");
}
}
// This code is contributed by AnkitRai01
Javascript
输出:
Given circles are orthogonal.
如果您希望与专家一起参加现场课程,请参阅DSA 现场工作专业课程和学生竞争性编程现场课程。