在本文中,我们将了解如何在 TCL 中使用程序。过程就像我们在任何其他编程语言(如 C、 Java、 Python等)中使用的函数一样。就像函数一样,过程接受参数并返回一些值。让我们通过一个简单的程序来调用过程来逐步打印两个数字的加、减、乘、除和模。
先决条件——
如果您想了解 TCL 脚本的基础知识,请阅读这篇文章 https://www.geeksforgeeks.org/basics-of-ns2-and-otcltcl-script/。
概述:
我们将通过逐块探索代码来尝试理解 TCL 中过程的语法。此外,我们还将查看 C 编程中过程的语法,以便更好地进行比较和理解。
TCL脚本演示程序:
让我们讨论以下步骤。
步骤1 :
让我们首先定义我们的程序。我们使用proc关键字来做到这一点。创建过程addnumbers {} 、 sub numbers {} 、 mulnumbers {} 、 divnumbers {}和modnumbers {}分别用于计算两个数字的和、差、乘积、除法和模数。
TCL script -
//Addition
proc addnumbers{ a b } {
return [expr $a + $b] }
//Subtraction
proc subnumbers{ a b } {
return [expr $a - $b] }
//Multiplication
proc mulnumbers{ a b } {
return [expr $a * $b] }
//Division
proc divnumbers{ a b } {
return [expr $a / $b] }
//Modulus
proc modnumbers{ a b } {
return [expr $a % $b] }
如上所示,语法可以概括如下。
proc procedurename {arguments} {
#body of the procedure
}
笔记 –
语法必须完全如上所示。如果忽略空格或在新行中键入左花括号,结果将是错误。还要注意像函数这样的过程可能有也可能没有返回类型。现在让我们看看上面这组函数在 C 编程中的样子。
C
//Addition
int addnumbers(int a, int b) {
return a + b; }
//Subtraction
int subnumbers(int a, int b) {
return a - b; }
//Multiplication
int mulnumbers(int a, int b) {
return a * b; }
//Division
float divnumbers(float a, float b) {
return a / b; }
//Modulus
int modnumbers(int a, int b) {
return a % b; }
第2步 :
下一步是使用 get 读取两个数字 a 和 b。
puts "Enter the first number"
gets stdin a
puts "Enter the second number"
gets stdin b
第 3 步:
最后一步是打印所有需要的值。在这里,我们还将查看 C 编程中的语法,以了解我们如何在 TCL 中调用函数。
puts "The sum of two numbers is [addnumbers $a $b]"
puts "The difference between the two numbers is [subnumbers $a $b]"
puts "The product of the two numbers is [subnumbers $a $b]"
puts "The division of the two numbers is [divnumbers $a $b]"
puts "The modulo of the two numbers is [modnumbers $a $b]"
因此,我们在上面看到调用过程的语法如下所示。
[procedurename $argument1 $argument2 .....]
现在让我们比较一下 C 编程中调用函数的语法。
functionname(argument1,argument2,.....)
第四步 :
最后,我们查看带有输出的整个代码,如下所示。
代码 –
//Addition
proc addnumbers {a b} {
return [expr $a+$b]
}
//Subtraction
proc subnumbers {a b} {
return [expr $a-$b]
}
//Multiplication
proc mulnumbers {a b} {
return [expr $a*$b]
}
//Division
proc divnumbers {a b} {
return [expr $a/$b]
}
//Modulus
proc modnumbers {a b} {
return [expr $a%$b]
}
//Input-1
puts "Enter the first number"
gets stdin a
//Input-2
puts "Enter the second number"
gets stdin b
//called procedures
puts "The sum of two numbers is [addnumbers $a $b]"
puts "The difference between the two numbers is [subnumbers $a $b]"
puts "The product of the two numbers is [subnumbers $a $b]"
puts "The division of the two numbers is [divnumbers $a $b]"
puts "The modulo of the two numbers is [modnumbers $a $b]"
输出 :
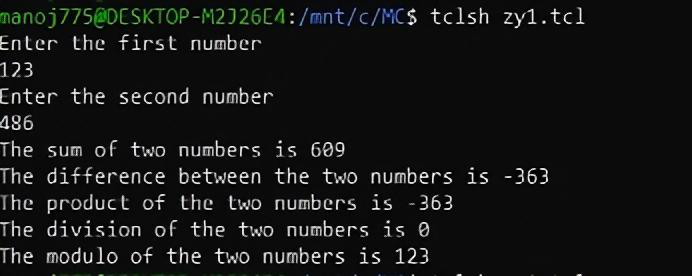
a=123 和 b=486 的输出