本文介绍了如何删除二进制文件的内容。
给定一个包含学生记录的二进制文件,任务是删除指定学生的记录。如果没有这样的学生的记录,则打印“找不到记录”。
例子:
Input:
roll no: 1
Output
The deleted record is
roll no: 1
name: vinay
record successfully deleted
Input:
roll no: 2
Output:
record not found
方法:
在此示例中,要从用户那里获取要删除其记录的学生的现有卷号,我们将创建一个新文件,在该文件中,我们将写入第一个文件的所有记录,但要删除的记录除外,然后将其删除第一个文件,并使用第一个文件的名称重命名新文件
以下是执行此操作的各个步骤:
- 步骤1:在“ he.dat”阅读模式下打开要从中删除记录的文件
- 步骤2:在此处“ temp.dat”下以写入模式打开要向其写入新内容的文件
- 步骤3:读取文件并将记录卷号与要删除的记录卷进行比较
- 步骤4:如果在读取过程中存在要删除的卷号,则显示该卷号,否则将记录写入temp.dat中
- 步骤5 :最后,在读取完整文件后,删除文件“ he.dat”,并将新文件“ temp.dat”重命名为“ he.dat”
- 步骤6:如果文件记录中存在,则打印记录并成功删除打印记录
- 步骤7:如果卷号不存在,则打印“找不到记录”。
使用的标准库函数:
// to remove the file
remove("name_of_file");
// to rename file1 as file2
rename("name-of_file1", "name_of_file2");
下面是上述方法的实现:
// C++ program to delete the record
// of a binary file
#include
using namespace std;
class abc {
int roll;
char name[20];
public:
void deleteit(int);
void testcase1();
void testcase2();
void putdata();
};
// Code to display the data of the
// data of the object
void abc::putdata()
{
cout << "roll no: ";
cout << roll;
cout << "\nname: ";
cout << name;
}
// code to delete
// the content of the binary file
void abc::deleteit(int rno)
{
int pos, flag = 0;
ifstream ifs;
ifs.open("he.dat", ios::in | ios::binary);
ofstream ofs;
ofs.open("temp.dat", ios::out | ios::binary);
while (!ifs.eof()) {
ifs.read((char*)this, sizeof(abc));
// if(ifs)checks the buffer record in the file
if (ifs) {
// comparing the roll no with
// roll no of record to be deleted
if (rno == roll) {
flag = 1;
cout << "The deleted record is \n";
// display the record
putdata();
}
else {
// copy the record of "he" file to "temp" file
ofs.write((char*)this, sizeof(abc));
}
}
}
ofs.close();
ifs.close();
// delete the old file
remove("he.dat");
// rename new file to the older file
rename("temp.dat", "he.dat");
if (flag == 1)
cout << "\nrecord successfully deleted \n";
else
cout << "\nrecord not found \n";
}
// Sample input 1
void abc::testcase1()
{
int rno;
// roll no to be searched
rno = 1;
// call deleteit function
// with the roll no. of record to be deleted
deleteit(rno);
}
// Sample input 2
void abc::testcase2()
{
int rno;
// roll no to be searched
rno = 4;
// call deleteit function
// with the roll no of record to be deleted
deleteit(rno);
}
// Driver code
int main()
{
abc s;
// sample case 1
s.testcase1();
// sample case 2
s.testcase2();
return 0;
}
输出:
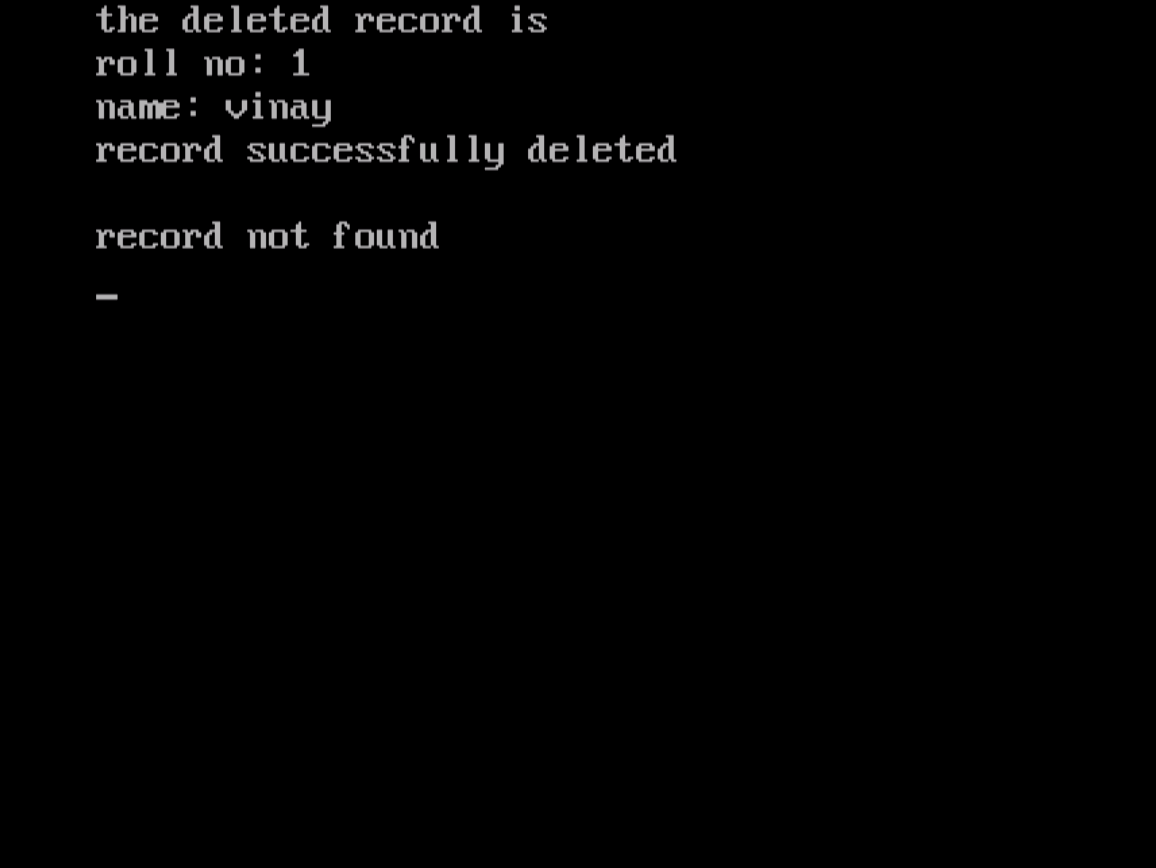
删除文件记录
想要从精选的最佳视频中学习并解决问题,请查看有关从基础到高级C++的C++基础课程以及有关语言和STL的C++ STL课程。要完成从学习语言到DS Algo等的更多准备工作,请参阅“完整面试准备课程” 。