Python的贪吃蛇游戏——使用 Pygame 模块
贪吃蛇游戏是有史以来最受欢迎的街机游戏之一。在这个游戏中,玩家的主要目标是在不撞墙或不撞墙的情况下抓住最大数量的水果。在学习Python或 Pygame 时,可以将创建蛇游戏视为一项挑战。这是每个新手程序员都应该接受的最好的初学者友好项目之一。学习构建视频游戏是一种有趣而有趣的学习。
我们将使用Pygame来创建这个蛇游戏。 Pygame是一个开源库,专为制作视频游戏而设计。它具有内置的图形和声音库。它也是初学者友好的和跨平台的。
安装
要安装 Pygame,您需要打开终端或命令提示符并键入以下命令:
pip install pygame
安装 Pygame 后,我们就可以创建我们很酷的蛇游戏了。
使用 Pygame 创建贪吃蛇游戏的分步方法:
第 1 步:首先,我们正在导入必要的库。
- 之后,我们将定义游戏将在其中运行的窗口的宽度和高度。
- 并以 RGB 格式定义我们将在游戏中用于显示文本的颜色。
Python3
# importing libraries
import pygame
import time
import random
snake_speed = 15
# Window size
window_x = 720
window_y = 480
# defining colors
black = pygame.Color(0, 0, 0)
white = pygame.Color(255, 255, 255)
red = pygame.Color(255, 0, 0)
green = pygame.Color(0, 255, 0)
blue = pygame.Color(0, 0, 255)
Python3
# Initialising pygame
pygame.init()
# Initialise game window
pygame.display.set_caption('GeeksforGeeks Snakes')
game_window = pygame.display.set_mode((window_x, window_y))
# FPS (frames per second) controller
fps = pygame.time.Clock()
Python3
# defining snake default position
snake_position = [100, 50]
# defining first 4 blocks of snake
# body
snake_body = [ [100, 50],
[90, 50],
[80, 50],
[70, 50]
]
# fruit position
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
# setting default snake direction
# towards right
direction = 'RIGHT'
change_to = direction
Python3
# initial score
score = 0
# displaying Score function
def show_score(choice, color, font, size):
# creating font object score_font
score_font = pygame.font.SysFont(font, size)
# create the display surface object
# score_surface
score_surface = score_font.render('Score : ' + str(score), True, color)
# create a rectangular object for the
# text surface object
score_rect = score_surface.get_rect()
# displaying text
game_window.blit(score_surface, score_rect)
Python3
# game over function
def game_over():
# creating font object my_font
my_font = pygame.font.SysFont('times new roman', 50)
# creating a text surface on which text
# will be drawn
game_over_surface = my_font.render('Your Score is : ' + str(score), True, red)
# create a rectangular object for the text
# surface object
game_over_rect = game_over_surface.get_rect()
# setting position of the text
game_over_rect.midtop = (window_x/2, window_y/4)
# blit wil draw the text on screen
game_window.blit(game_over_surface, game_over_rect)
pygame.display.flip()
# after 2 seconds we will quit the
# program
time.sleep(2)
# deactivating pygame library
pygame.quit()
# quit the program
quit()
Python3
# Main Function
while True:
# handling key events
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
change_to = 'UP'
if event.key == pygame.K_DOWN:
change_to = 'DOWN'
if event.key == pygame.K_LEFT:
change_to = 'LEFT'
if event.key == pygame.K_RIGHT:
change_to = 'RIGHT'
# If two keys pressed simultaneously
# we don't want snake to move into two directions
# simultaneously
if change_to == 'UP' and direction != 'DOWN':
direction = 'UP'
if change_to == 'DOWN' and direction != 'UP':
direction = 'DOWN'
if change_to == 'LEFT' and direction != 'RIGHT':
direction = 'LEFT'
if change_to == 'RIGHT' and direction != 'LEFT':
direction = 'RIGHT'
# Moving the snake
if direction == 'UP':
snake_position[1] -= 10
if direction == 'DOWN':
snake_position[1] += 10
if direction == 'LEFT':
snake_position[0] -= 10
if direction == 'RIGHT':
snake_position[0] += 10
# Snake body growing mechanism
# if fruits and snakes collide then scores will be
# incremented by 10
snake_body.insert(0, list(snake_position))
if snake_position[0] == fruit_position[0] and snake_position[1] == fruit_position[1]:
score += 10
fruit_spawn = False
else:
snake_body.pop()
if not fruit_spawn:
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
game_window.fill(black)
for pos in snake_body:
pygame.draw.rect(game_window, green, pygame.Rect(
pos[0], pos[1], 10, 10))
pygame.draw.rect(game_window, white, pygame.Rect(
fruit_position[0], fruit_position[1], 10, 10))
# Game Over conditions
if snake_position[0] < 0 or snake_position[0] > window_x-10:
game_over()
if snake_position[1] < 0 or snake_position[1] > window_y-10:
game_over()
# Touching the snake body
for block in snake_body[1:]:
if snake_position[0] == block[0] and snake_position[1] == block[1]:
game_over()
# displaying score countinuously
show_score(1, white, 'times new roman', 20)
# Refresh game screen
pygame.display.update()
# Frame Per Second /Refres Rate
fps.tick(snake_speed)
Python3
# importing libraries
import pygame
import time
import random
snake_speed = 15
# Window size
window_x = 720
window_y = 480
# defining colors
black = pygame.Color(0, 0, 0)
white = pygame.Color(255, 255, 255)
red = pygame.Color(255, 0, 0)
green = pygame.Color(0, 255, 0)
blue = pygame.Color(0, 0, 255)
# Initialising pygame
pygame.init()
# Initialise game window
pygame.display.set_caption('GeeksforGeeks Snakes')
game_window = pygame.display.set_mode((window_x, window_y))
# FPS (frames per second) controller
fps = pygame.time.Clock()
# defining snake default position
snake_position = [100, 50]
# defining first 4 blocks of snake body
snake_body = [[100, 50],
[90, 50],
[80, 50],
[70, 50]
]
# fruit position
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
# setting default snake direction towards
# right
direction = 'RIGHT'
change_to = direction
# initial score
score = 0
# displaying Score function
def show_score(choice, color, font, size):
# creating font object score_font
score_font = pygame.font.SysFont(font, size)
# create the display surface object
# score_surface
score_surface = score_font.render('Score : ' + str(score), True, color)
# create a rectangular object for the text
# surface object
score_rect = score_surface.get_rect()
# displaying text
game_window.blit(score_surface, score_rect)
# game over function
def game_over():
# creating font object my_font
my_font = pygame.font.SysFont('times new roman', 50)
# creating a text surface on which text
# will be drawn
game_over_surface = my_font.render(
'Your Score is : ' + str(score), True, red)
# create a rectangular object for the text
# surface object
game_over_rect = game_over_surface.get_rect()
# setting position of the text
game_over_rect.midtop = (window_x/2, window_y/4)
# blit wil draw the text on screen
game_window.blit(game_over_surface, game_over_rect)
pygame.display.flip()
# after 2 seconds we will quit the program
time.sleep(2)
# deactivating pygame library
pygame.quit()
# quit the program
quit()
# Main Function
while True:
# handling key events
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
change_to = 'UP'
if event.key == pygame.K_DOWN:
change_to = 'DOWN'
if event.key == pygame.K_LEFT:
change_to = 'LEFT'
if event.key == pygame.K_RIGHT:
change_to = 'RIGHT'
# If two keys pressed simultaneously
# we don't want snake to move into two
# directions simultaneously
if change_to == 'UP' and direction != 'DOWN':
direction = 'UP'
if change_to == 'DOWN' and direction != 'UP':
direction = 'DOWN'
if change_to == 'LEFT' and direction != 'RIGHT':
direction = 'LEFT'
if change_to == 'RIGHT' and direction != 'LEFT':
direction = 'RIGHT'
# Moving the snake
if direction == 'UP':
snake_position[1] -= 10
if direction == 'DOWN':
snake_position[1] += 10
if direction == 'LEFT':
snake_position[0] -= 10
if direction == 'RIGHT':
snake_position[0] += 10
# Snake body growing mechanism
# if fruits and snakes collide then scores
# will be incremented by 10
snake_body.insert(0, list(snake_position))
if snake_position[0] == fruit_position[0] and snake_position[1] == fruit_position[1]:
score += 10
fruit_spawn = False
else:
snake_body.pop()
if not fruit_spawn:
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
game_window.fill(black)
for pos in snake_body:
pygame.draw.rect(game_window, green,
pygame.Rect(pos[0], pos[1], 10, 10))
pygame.draw.rect(game_window, white, pygame.Rect(
fruit_position[0], fruit_position[1], 10, 10))
# Game Over conditions
if snake_position[0] < 0 or snake_position[0] > window_x-10:
game_over()
if snake_position[1] < 0 or snake_position[1] > window_y-10:
game_over()
# Touching the snake body
for block in snake_body[1:]:
if snake_position[0] == block[0] and snake_position[1] == block[1]:
game_over()
# displaying score countinuously
show_score(1, white, 'times new roman', 20)
# Refresh game screen
pygame.display.update()
# Frame Per Second /Refres Rate
fps.tick(snake_speed)
第 2 步:导入库后,我们需要使用pygame.init()方法初始化 Pygame。
- 使用上一步中定义的宽度和高度创建一个游戏窗口。
- 这里pygame.time.Clock()将在游戏的主要逻辑中进一步用于改变蛇的速度。
蟒蛇3
# Initialising pygame
pygame.init()
# Initialise game window
pygame.display.set_caption('GeeksforGeeks Snakes')
game_window = pygame.display.set_mode((window_x, window_y))
# FPS (frames per second) controller
fps = pygame.time.Clock()
第 3 步:初始化蛇的位置及其大小。
- 初始化蛇位置后,在定义的高度和宽度的任意位置随机初始化水果位置。
- 通过将方向设置为 RIGHT,我们确保每当用户运行程序/游戏时,蛇必须向右移动到屏幕上。
蟒蛇3
# defining snake default position
snake_position = [100, 50]
# defining first 4 blocks of snake
# body
snake_body = [ [100, 50],
[90, 50],
[80, 50],
[70, 50]
]
# fruit position
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
# setting default snake direction
# towards right
direction = 'RIGHT'
change_to = direction
第 4 步:创建一个函数来显示玩家的得分。
- 在这个函数,首先我们要创建一个字体对象,即字体颜色会出现在这里。
- 然后我们使用渲染来创建一个背景表面,每当我们的分数更新时,我们就会改变它。
- 为文本表面对象创建一个矩形对象(文本将在此处刷新)
- 然后,我们使用blit显示我们的分数。 blit需要两个参数screen.blit(background,(x,y))
蟒蛇3
# initial score
score = 0
# displaying Score function
def show_score(choice, color, font, size):
# creating font object score_font
score_font = pygame.font.SysFont(font, size)
# create the display surface object
# score_surface
score_surface = score_font.render('Score : ' + str(score), True, color)
# create a rectangular object for the
# text surface object
score_rect = score_surface.get_rect()
# displaying text
game_window.blit(score_surface, score_rect)
第 5 步:现在创建一个游戏结束函数,该函数将代表蛇被墙壁或自身击中后的分数。
- 在第一行,我们创建了一个字体对象来显示乐谱。
- 然后我们创建文本表面来渲染乐谱。
- 之后,我们将设置文本在可播放区域中间的位置。
- 使用blit显示分数并通过使用 flip() 更新表面来更新分数。
- 我们使用 sleep(2) 在使用 quit() 关闭窗口之前等待 2 秒。
蟒蛇3
# game over function
def game_over():
# creating font object my_font
my_font = pygame.font.SysFont('times new roman', 50)
# creating a text surface on which text
# will be drawn
game_over_surface = my_font.render('Your Score is : ' + str(score), True, red)
# create a rectangular object for the text
# surface object
game_over_rect = game_over_surface.get_rect()
# setting position of the text
game_over_rect.midtop = (window_x/2, window_y/4)
# blit wil draw the text on screen
game_window.blit(game_over_surface, game_over_rect)
pygame.display.flip()
# after 2 seconds we will quit the
# program
time.sleep(2)
# deactivating pygame library
pygame.quit()
# quit the program
quit()
第 6 步:现在我们将创建我们的主要函数,它将执行以下操作:
- 我们将验证负责蛇移动的密钥,然后我们将创建一个特殊条件,即不允许蛇立即向相反方向移动。
- 在那之后,如果蛇和水果发生碰撞,我们将把分数增加 10,新的水果将被跨越。
- 在那之后,我们正在检查蛇是否被墙击中。如果一条蛇撞到墙,我们将调用 game over函数。
- 如果蛇撞到自己,游戏结束函数将被调用。
- 最后,我们将使用之前创建的 show_score函数显示分数。
蟒蛇3
# Main Function
while True:
# handling key events
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
change_to = 'UP'
if event.key == pygame.K_DOWN:
change_to = 'DOWN'
if event.key == pygame.K_LEFT:
change_to = 'LEFT'
if event.key == pygame.K_RIGHT:
change_to = 'RIGHT'
# If two keys pressed simultaneously
# we don't want snake to move into two directions
# simultaneously
if change_to == 'UP' and direction != 'DOWN':
direction = 'UP'
if change_to == 'DOWN' and direction != 'UP':
direction = 'DOWN'
if change_to == 'LEFT' and direction != 'RIGHT':
direction = 'LEFT'
if change_to == 'RIGHT' and direction != 'LEFT':
direction = 'RIGHT'
# Moving the snake
if direction == 'UP':
snake_position[1] -= 10
if direction == 'DOWN':
snake_position[1] += 10
if direction == 'LEFT':
snake_position[0] -= 10
if direction == 'RIGHT':
snake_position[0] += 10
# Snake body growing mechanism
# if fruits and snakes collide then scores will be
# incremented by 10
snake_body.insert(0, list(snake_position))
if snake_position[0] == fruit_position[0] and snake_position[1] == fruit_position[1]:
score += 10
fruit_spawn = False
else:
snake_body.pop()
if not fruit_spawn:
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
game_window.fill(black)
for pos in snake_body:
pygame.draw.rect(game_window, green, pygame.Rect(
pos[0], pos[1], 10, 10))
pygame.draw.rect(game_window, white, pygame.Rect(
fruit_position[0], fruit_position[1], 10, 10))
# Game Over conditions
if snake_position[0] < 0 or snake_position[0] > window_x-10:
game_over()
if snake_position[1] < 0 or snake_position[1] > window_y-10:
game_over()
# Touching the snake body
for block in snake_body[1:]:
if snake_position[0] == block[0] and snake_position[1] == block[1]:
game_over()
# displaying score countinuously
show_score(1, white, 'times new roman', 20)
# Refresh game screen
pygame.display.update()
# Frame Per Second /Refres Rate
fps.tick(snake_speed)
下面是实现
蟒蛇3
# importing libraries
import pygame
import time
import random
snake_speed = 15
# Window size
window_x = 720
window_y = 480
# defining colors
black = pygame.Color(0, 0, 0)
white = pygame.Color(255, 255, 255)
red = pygame.Color(255, 0, 0)
green = pygame.Color(0, 255, 0)
blue = pygame.Color(0, 0, 255)
# Initialising pygame
pygame.init()
# Initialise game window
pygame.display.set_caption('GeeksforGeeks Snakes')
game_window = pygame.display.set_mode((window_x, window_y))
# FPS (frames per second) controller
fps = pygame.time.Clock()
# defining snake default position
snake_position = [100, 50]
# defining first 4 blocks of snake body
snake_body = [[100, 50],
[90, 50],
[80, 50],
[70, 50]
]
# fruit position
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
# setting default snake direction towards
# right
direction = 'RIGHT'
change_to = direction
# initial score
score = 0
# displaying Score function
def show_score(choice, color, font, size):
# creating font object score_font
score_font = pygame.font.SysFont(font, size)
# create the display surface object
# score_surface
score_surface = score_font.render('Score : ' + str(score), True, color)
# create a rectangular object for the text
# surface object
score_rect = score_surface.get_rect()
# displaying text
game_window.blit(score_surface, score_rect)
# game over function
def game_over():
# creating font object my_font
my_font = pygame.font.SysFont('times new roman', 50)
# creating a text surface on which text
# will be drawn
game_over_surface = my_font.render(
'Your Score is : ' + str(score), True, red)
# create a rectangular object for the text
# surface object
game_over_rect = game_over_surface.get_rect()
# setting position of the text
game_over_rect.midtop = (window_x/2, window_y/4)
# blit wil draw the text on screen
game_window.blit(game_over_surface, game_over_rect)
pygame.display.flip()
# after 2 seconds we will quit the program
time.sleep(2)
# deactivating pygame library
pygame.quit()
# quit the program
quit()
# Main Function
while True:
# handling key events
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
change_to = 'UP'
if event.key == pygame.K_DOWN:
change_to = 'DOWN'
if event.key == pygame.K_LEFT:
change_to = 'LEFT'
if event.key == pygame.K_RIGHT:
change_to = 'RIGHT'
# If two keys pressed simultaneously
# we don't want snake to move into two
# directions simultaneously
if change_to == 'UP' and direction != 'DOWN':
direction = 'UP'
if change_to == 'DOWN' and direction != 'UP':
direction = 'DOWN'
if change_to == 'LEFT' and direction != 'RIGHT':
direction = 'LEFT'
if change_to == 'RIGHT' and direction != 'LEFT':
direction = 'RIGHT'
# Moving the snake
if direction == 'UP':
snake_position[1] -= 10
if direction == 'DOWN':
snake_position[1] += 10
if direction == 'LEFT':
snake_position[0] -= 10
if direction == 'RIGHT':
snake_position[0] += 10
# Snake body growing mechanism
# if fruits and snakes collide then scores
# will be incremented by 10
snake_body.insert(0, list(snake_position))
if snake_position[0] == fruit_position[0] and snake_position[1] == fruit_position[1]:
score += 10
fruit_spawn = False
else:
snake_body.pop()
if not fruit_spawn:
fruit_position = [random.randrange(1, (window_x//10)) * 10,
random.randrange(1, (window_y//10)) * 10]
fruit_spawn = True
game_window.fill(black)
for pos in snake_body:
pygame.draw.rect(game_window, green,
pygame.Rect(pos[0], pos[1], 10, 10))
pygame.draw.rect(game_window, white, pygame.Rect(
fruit_position[0], fruit_position[1], 10, 10))
# Game Over conditions
if snake_position[0] < 0 or snake_position[0] > window_x-10:
game_over()
if snake_position[1] < 0 or snake_position[1] > window_y-10:
game_over()
# Touching the snake body
for block in snake_body[1:]:
if snake_position[0] == block[0] and snake_position[1] == block[1]:
game_over()
# displaying score countinuously
show_score(1, white, 'times new roman', 20)
# Refresh game screen
pygame.display.update()
# Frame Per Second /Refres Rate
fps.tick(snake_speed)
输出:
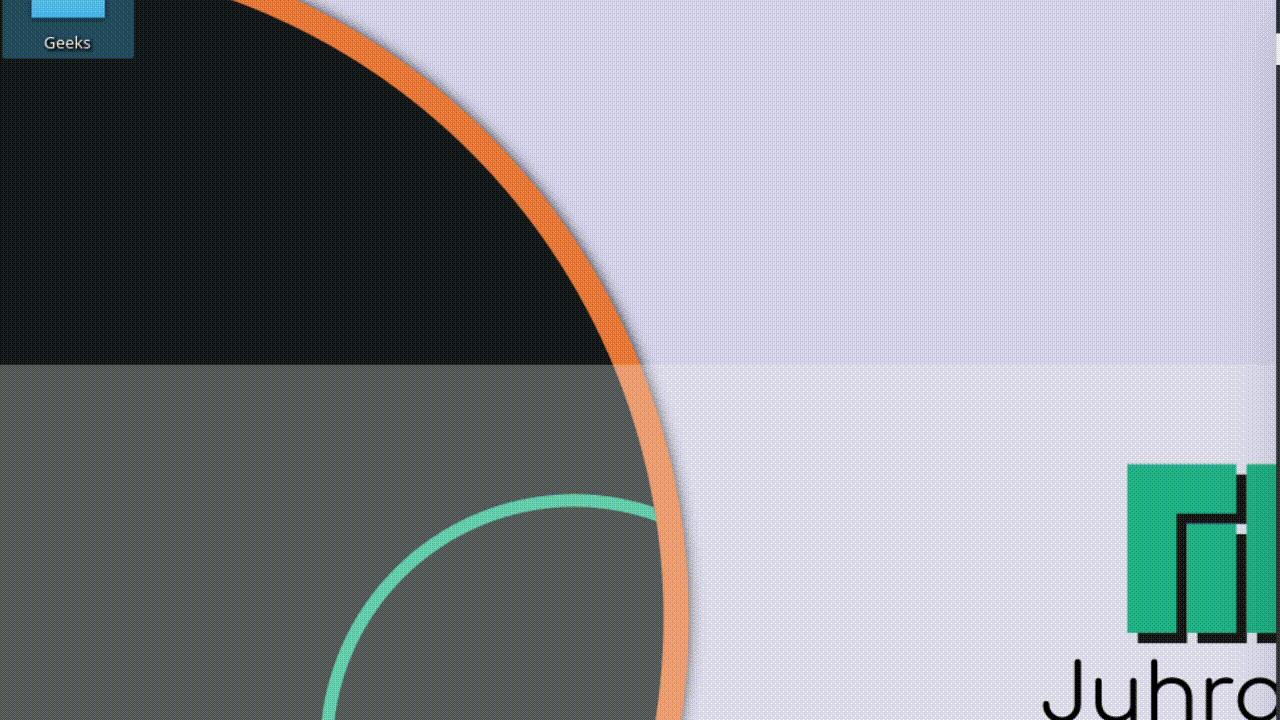
Snake 使用 Pygame